Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial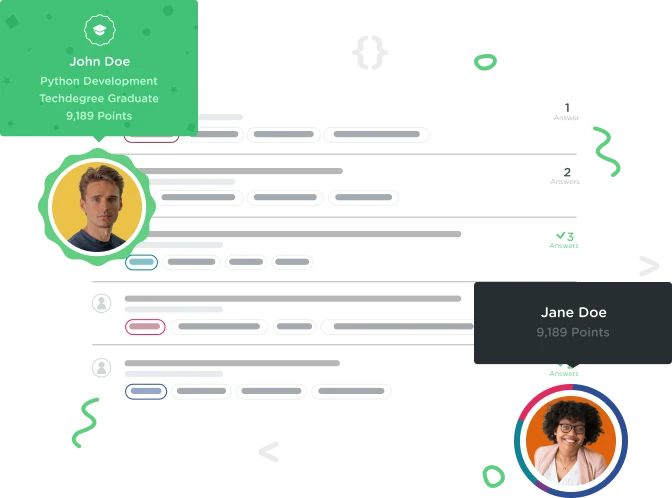

seongho kang
3,877 PointsI need your help to solve this Task.
I don't get this is not working well. Which one did i miss for solving this task?
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let p = e.target.parentNode;
let prevP = p.previousElementSibling;
let li = p.parentNode;
if (prevP) {
li.insertBefore(p, prevP);
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
2 Answers
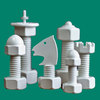
Steven Parker
231,269 PointsHere's some hints:
- you want to target the sibling of the button, not the sibling of the button's parent.
- you don't need to move any element positions
- just give the sibling paragraph a new class attribute ("
highlight
")

Daniel Baker
15,369 Pointsvar list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let p = e.target.parentNode; // This will actually be the <li>, the <p>is a sibling not the parent
let prevP = p.previousElementSibling; //this will attempt to be the previous sibling of <li>
let li = p.parentNode; //this will be the <ul>
if (prevP) {
li.insertBefore(p, prevP);
}
}
});
You only need to do two steps.
- Get the
previousElementSibling
of the button - Add the
className
of"highlight"
to that sibling
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let sibling = e.target.previousElementSibling;
sibling.className = "highlight";
}
});