Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial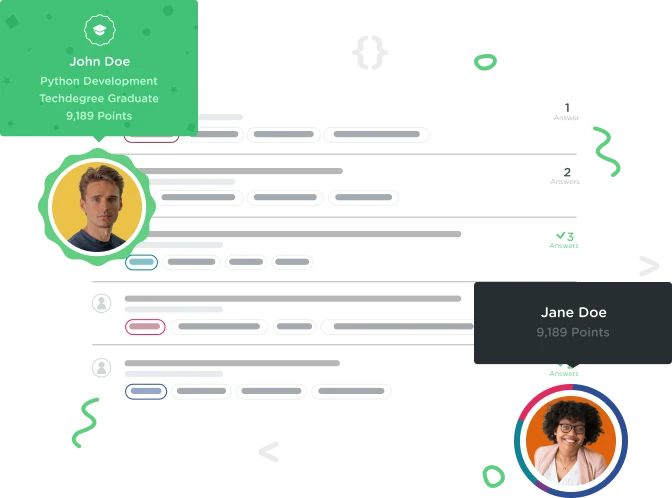

Jonathon Mohon
3,165 PointsI noticed that my function is quite different from everyone else's but it works. Is there a flaw I don't see however?
When I capture the player's move and call my function, inside my function I unpack the player tuple instead of using indexes/array like everyone else. I was just wondering if this way is acceptable i guess. (update_location and test_move are what i'm talking about)
import random
CELLS = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_start_locations():
# monster = random
loc_monster = random.choice(CELLS)
# door = random
loc_door = random.choice(CELLS)
# player start location= random
player_start = random.choice(CELLS)
# if monster, door, or start are the same, do it again
if loc_door == player_start:
get_start_locations()
elif player_start == loc_monster:
get_start_locations()
elif loc_monster == loc_door:
get_start_locations()
else:
return loc_monster, loc_door, player_start
def update_location(tpl, char):
first_coord, second_coord = tpl
if char == 'U':
first_coord -= 1
elif char == 'D':
first_coord += 1
elif char == 'R':
second_coord += 1
elif char == 'L':
second_coord -= 1
new_coord = (first_coord, second_coord)
if test_move(new_coord):
return new_coord
else:
return tpl
def test_move(tpl):
first_coord, second_coord = tpl
if first_coord not in range(0, 3):
return False
elif second_coord not in range(0, 3):
return False
else:
return True
monster, door, player = get_start_locations()
# print(monster)
# print(door)
# print(player)
while True:
print("Welcome to the dungeon!")
print("You're currently in room {}".format(player)) # fill in with player current position
print("You can move 'U' up, 'D' down, 'R' right, 'L' left")
print("Enter QUIT to quit.")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
# if it's a good move, change the player's position
new_player = update_location(player, move)
if new_player != player:
player = new_player
# if it's a bad move, don't change anything, display msg
else:
print("Can't move in the {} direction you're at the edge".format(move))
# if the player's new position is the door, they win
if player == door:
print("You found the door, you win!")
break
# if the player's new position is the monster, they lose
elif player == monster:
print("You lose! Eaten by a monster")
break
# otherwise, continue
else:
continue
1 Answer
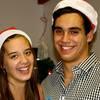
Cody Te Awa
8,820 PointsThat way is perfect Jonathon, and in my opinion even a little bit better. Allows the code to be slightly more readable and a little more pythonic! Great Job Jonathon! Keep up the good work! Happy coding :)