Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial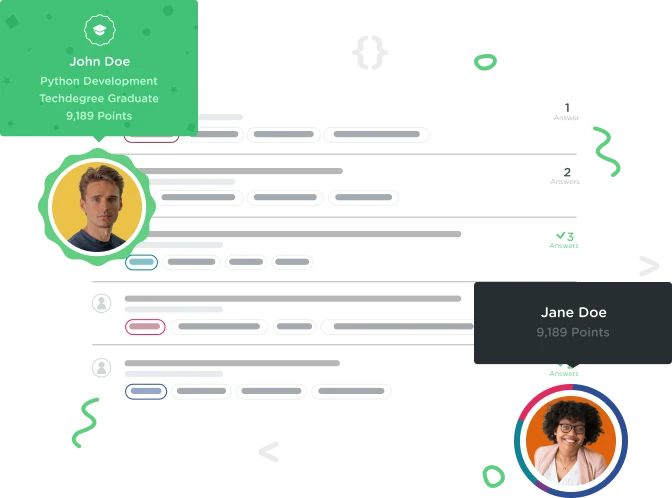

NICOLE REBENTISCH
3,612 PointsI prefer the alert to pop up as soon as a non-number is typed. Is there a more concise way than how I coded it?
The example given by Dave McFarland in the Practice If and Else exercise doesn't pop up an alert until after both values are entered, but I prefer the alert to pop up as soon as a non-number is typed (or if "cancel" is clicked). I came up with the code below to address that, but is there a shorter way to achieve the same result?
// declare program variables
let num1;
let num2 = null;
let failMessage = 0;
let message;
// announce the program
alert("Let's do some math!");
// collect numeric input
num1 = prompt("Please type a number");
num1 = parseFloat(num1);
if (isNaN(num1)){
alert( "The value you typed is not a number. Reload and try again.");
failMessage = 1;
}
else if (num1 !== NaN) {
num2 = prompt("Please type another number");
num2 = parseFloat(num2);
}
if (num2 === 0){
alert("The second number is 0. You can't divide by zero. Reload and try again.");
failMessage = 1;
}
else if ( (isNaN(num2)) && (num1 !== NaN)){
alert( "The value you typed is not a number. Reload and try again.");
failMessage = 1;
}
// build an HTML message
message = `<h1>Math with the numbers ${num1} and ${num2}</h1>
${num1} + ${num2} = ${num1 + num2}
<br>
${num1} * ${num2} = ${num1 * num2}
<br>
${num1} / ${num2} = ${num1 / num2}
<br>
${num1} - ${num2} = ${num1 - num2}`;
// write message to web page
if (failMessage === 0){
document.write(message);
}
2 Answers
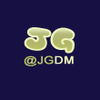
Jonathan Grieve
Treehouse Moderator 91,253 PointsTry calling parseFloat()
at the end of your prompt, so you don't have the pass the variables into it and call them so many times.
num1 = prompt("Please type a number").parseFloat();
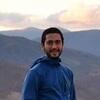
Martín Giavarini
4,036 PointsThis should avoid most cases of user input error:
do {
var num1 = prompt("Please type a number");
if (isNaN(num1)) {
alert("The value you typed is not a number. Try again.");
}
} while (isNaN(num1));
do {
var num2 = prompt("Please type another number");
if (+num2 === 0) {
alert("The second number is 0. You can't divide by zero. Try again.");
} else if (isNaN(num2)) {
alert("The value you typed is not a number. Try again.");
} else {
result = true;
}
} while (+num2 === 0 || isNaN(num2));
NICOLE REBENTISCH
3,612 PointsNICOLE REBENTISCH
3,612 PointsAdding
.parseFloat()
to the end of the line throws an error message. Thanks for the idea, though!Forrest Pollard
1,988 PointsForrest Pollard
1,988 Pointsthis should work