Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial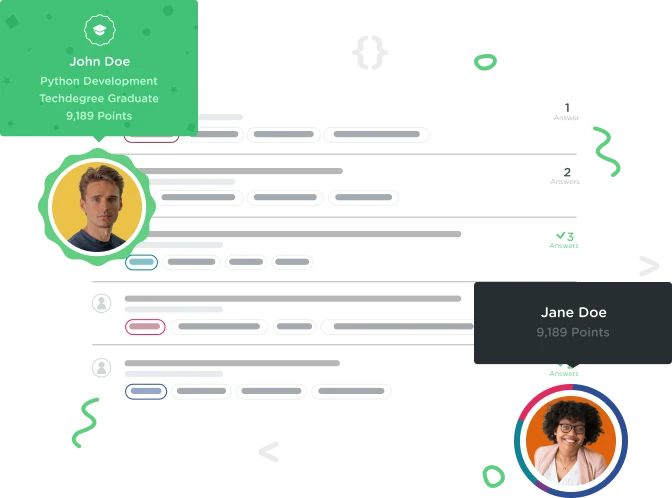
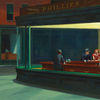
Anthony Grodowski
4,902 PointsI pretty much don't get why it's throwing an error at me...
In my workspace I get an output as expected but challange doesn't accept that code. What's wrong?
class Student:
def praise(self):
name = "Antek"
print("You look absolutely gorgeous {}!".format(name))
Also I don't quite get instances and self keyword. Can someone please explain me that(:?
2 Answers
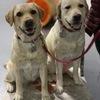
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Antoni,
The name
attribute belongs to the class Student
. You have moved it into your method.
When you put name
back to being at the class-level instead of method-level, you will see that you can no longer access it as just name
, because it isn't in your method's scope. This is where you use the self
keyword to access that variable.
For example:
class MyClass:
my_attribute = "hello, world"
def some_method(self):
print(self.my_attribute)
Observe that my_attribute
is outside the method some_method
and to access it we have to prefix it with self.
.
Instance
An instance is a concrete 'thing' made according to the description set out in the 'class'. Think of the class as being a recipe and an instance as being a particular cake made using that recipe.
self
A single class can make any number of instances, just like with a recipe we can make any number of cakes. Since the code in a particular class may end up in many objects, we need some way of referring to a particular instance of the class from inside the class description. This is where we use self
. It's hard to see with the example from the challenge because every instance of the class will be the same, but it might be more clear if we look at a simple example that gives every instance some unique value.
class Greeter:
def __init__(self, name):
self.name = name
def greet(self):
print("Hello, {}".format(self.name))
If some of the syntax above is unfamiliar, don't worry too much, just know that the __init__
method is what is used to create custom instances. Here we give it a name
value, and it'll make a custom Greeter with that name property inside it.
For example:
g1 = Greeter("alice")
g2 = Greeter("bob")
When we call the greet()
method on each instance, we'll get the correct name:
g1.greet() # "Hello, alice"
g2.greet() # "Hello, bob"
Observe that we are using self
in the greet
method to tell the class that we want to print the value that belongs to
that particular instance, not something that belongs to the class.
Hope that clears things up for you.
Cheers
Alex
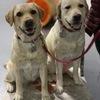
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Antoni,
I'm assuming you're referring to the use of self
inside the format
call. In which case, yes, you've got the right idea. Strictly, self
here is not a parameter, it's just part of the path to the variable. You're telling the format
method to look for the name
variable inside self
's namespace. Behind the scenes, Python replaces self
with the specific instance of the class.
Cheers
Alex
Anthony Grodowski
4,902 PointsAnthony Grodowski
4,902 PointsThank you very much! The cake example helped a lot. But still, even after moving the name attribute and adding self, the code isn't working hah (in the workspace it works perfectly after using "me = Student() print(me.praise())" )
What's wrong?
Anthony Grodowski
4,902 PointsAnthony Grodowski
4,902 PointsAlright, I've got this. I just had to replace print with return. I have one more question - "self" as a parameter is used to inform the method to take an argument from inside of the class?