Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial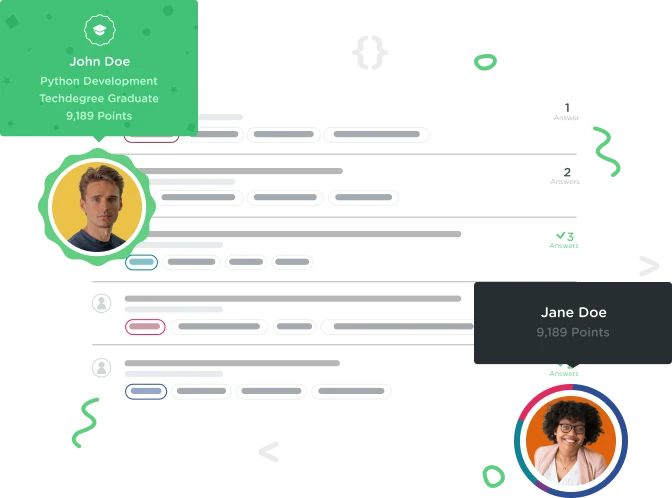

Victor Leschly Ørnby
Courses Plus Student 2,992 PointsI Problems with set method in computed properties
I having problems understanding the error message, and what I am doing wrong
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float{
get {
return (celsius * 1.8) + 32.0
}
set {
fahrenheit = newValue
celsius = ((fahrenheit-32)/1.8)
}
}
}
1 Answer
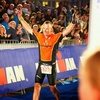
Steve Hunter
57,712 PointsHi Victor,
Getters and Setters are a bit weird and difficult to understand - they always confuse me! But that's quite easy!
The challenge wants you to create a getter for fahrenheit
that uses the existing celsius
value to calculate the conversion. The code provided at the start of the challenge does this. You just need to add the get
bit to it:
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get {
return (celsius * 1.8) + 32.0
}
set {
// do something
}
}
}
That just leaves the setter to complete.
Essentially, the setter will change the value of celsius
when the function is passed a value to fahrenheit
. So, the calculation needs to be reversed, i.e. subtract 32 then divide by 1.8. The calculation is performed on the newValue
which is the number passed into the function:
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get {
return (celsius * 1.8) + 32.0
}
set {
celsius = (newValue - 32) / 1.8
}
}
}
So, create am instance of Temperature and initialise it so celsius
has a value of 32.
Typing temp.celsius
will output 32.
Looking at temp.fahrenheit
will give you 89.59 using the get
part of the function.
To use the set
part, pass a value to fahrenheit
like:
Enter temp.fahrenheit = 90
Then, temp.celsius
will give you 15.55
So, you can influence each variable by changing the value of the other since they are mutually linked in that way.
Hope that helps,
Steve.