Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial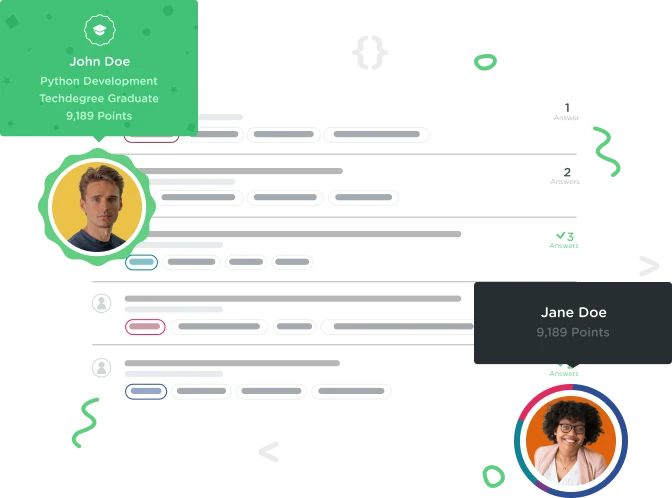

Abdullah Jassim
4,551 PointsI ran this code without .copy() and the code still works. What am I missing?
books = [
"Automate the Boring Stuff with Python: Practical Programming for Total Beginners - Al Sweigart",
"Python for Data Analysis",
"Fluent Python: Clear, Concise, and Effective Programming - Luciano Ramalho",
"Python for Kids: A Playful Introduction To Programming - Jason R. Briggs",
"Hello Web App: Learn How to Build a Web App - Tracy Osborn",
]
games = [
"Metal Gear Solid",
"Legend of Zelda",
"Mario Kart",
"Splinter Cell"
]
def display_wishlist(category, wishes):
print(category + ":")
suggested_book = wishes.pop()
print("======>", suggested_book, "<======")
for wish in wishes:
print("* " + wish)
print()
display_wishlist("Books", books)
display_wishlist("Games", games)
4 Answers
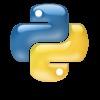
Viraj Deshaval
4,874 PointsI want to give you a reason why there is a need of copy(). Issue: When we try to loop through the list and remove the values using '. remove() or pop()' values gets shifted and due to which all values cannot be deleted.
Solution: we can use copy() to overcome this issue. This will be helpful to you. Let me know if you still require any help.

Abdullah Jassim
4,551 PointsThanks for the note. I understand how pop() modifies the list and hence all values will not be deleted. I wanted to see that in practice and hence I wrote the code above. However, when I ran the code several times, .pop() is supposed to give me a different of values for both games and books, but it doesnt. Which is why I am a bit confused as to what I may be doing wrong.
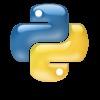
Viraj Deshaval
4,874 PointsOk let me give you an example. Suppose you remove values from the list without copy: items = ['a', 'b', 'c', 'd']
for item in items: items.remove(item)
print(items) Output : ['b', 'd']
This did not removed all values. Now I will use copy.
items = ['a', 'b', 'c', 'd']
for item in items.copy(): items.remove(item)
print(items) Output : []
So now all values were removed. What happened is we have copy of our items list which we will use for referencing the values of original items list and as we don't remove items from copied list values will not be shifted and list doesn't gets changed. Just remember copied list to reference original list. I hope I have cleared the doubt of yours. Let me know if you still require any help. Happy to help.

Kyler Aagard
1,331 PointsI’m new to this so I may be wrong, but I think you use .copy() because the list you started with is sort of a master list. So when you use .pop() you are changing the master list. So it is saying, of the things that are possible to be on this list, take one off. With .copy() it is saying, make a copy of the master list and display it with new parameters.

Michael Dioszeghy
2,412 PointsI'm new to this and may be wrong, but the code will work fine until you call the display_wishlist("Games", games) again. in the same program.
if you have something like this:
books = [ "Automate the Boring Stuff with Python: Practical Programming for Total Beginners - Al Sweigart", "Python for Data Analysis", "Fluent Python: Clear, Concise, and Effective Programming - Luciano Ramalho", "Python for Kids: A Playful Introduction To Programming - Jason R. Briggs", "Hello Web App: Learn How to Build a Web App - Tracy Osborn", ]
games = [ "Metal Gear Solid", "Legend of Zelda", "Mario Kart", "Splinter Cell" ]
def display_wishlist(category, wishes):
print(category + ":")
suggested_book = wishes.pop()
print("======>", suggested_book, "<======")
for wish in wishes:
print("* " + wish)
print()
display_wishlist("Books", books) display_wishlist("Games", games) display_wishlist("Games", games) display_wishlist("Games", games)
Your result becomes:
Books:
======> Hello Web App: Learn How to Build a Web App - Tracy Osborn <======
- Automate the Boring Stuff with Python: Practical Programming for Total Beginners - Al Sweigart
- Python for Data Analysis
- Fluent Python: Clear, Concise, and Effective Programming - Luciano Ramalho
- Python for Kids: A Playful Introduction To Programming - Jason R. Briggs
Games:
======> Splinter Cell <======
- Metal Gear Solid
- Legend of Zelda
- Mario Kart
Games:
======> Mario Kart <======
- Metal Gear Solid
- Legend of Zelda
Games:
======> Legend of Zelda <======
- Metal Gear Solid
This is why the .copy() function is important because otherwise it will keep removing the last item from the list and changing it.

Andrejs Usacs
2,308 PointsHi Abdullah Jassim.
In the video Craig duplicated the last code """display_wishlist("Games", games)""" If in the end you have: """ display_wishlist("Books", books) display_wishlist("Games", games) display_wishlist("Games", games) """ One of the suggestions from games list will be deleted.
Istvan Nonn
2,092 PointsIstvan Nonn
2,092 PointsWhat I think is that your code is written in a text editor and saved in a .py file. And for testing the code you are using a terminal. In this case I can imagine the result will be the same every time you test your code.