Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial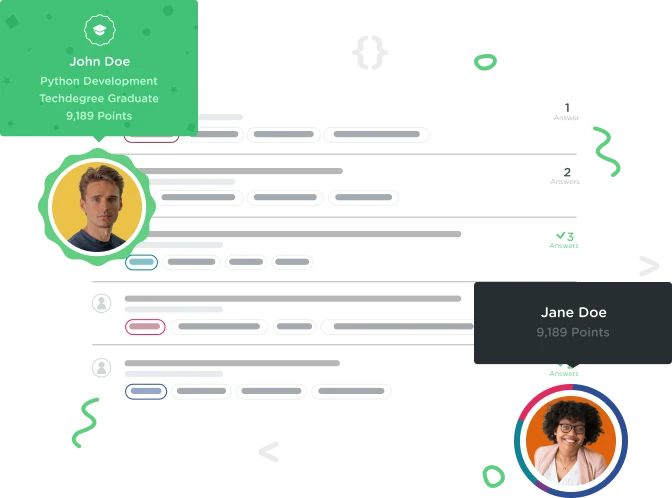
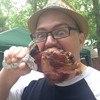
Jake Schmidt
1,536 PointsI realize that its printing the whole range to the console each time through the loop instead of each number, but why?
But why???
var num = ' ';
for ( var i = 4; i <= 156; i += 1 ) {
num += i + ' ' ;
console.log(num);
}
2 Answers

Brian Holland
3,508 PointsIt's not actually not printing the whole range every time. It prints increasingly:
4
4 5
4 5 6
...
4 5 6 7 8..... 156
First of all, you don't actually need a 'num' variable at all. Just log each number once with console.log(i).
Second, javascript kind of 'bends' the typing of some variables when it can. Spot the difference:
str = ' '
num = []
obj = {}
Javascript assumes that you know that since num was declared as a string (i.e., with ' 's surrounding it), you mean you want to concatenate i to num as a string when you say num += i + ' '.
It's like the opposite of if I were to say console.log("i").
W3 Schools has a good page about it.
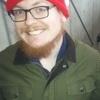
Christian Weatherford
8,848 PointsThe following code will print each number once, but each on its own line. No need for a "num" variable, just log out the value of "i".
for ( var i = 4; i <= 156; i += 1 ) {
console.log(i);
}
However, if you want it all on one line, I'd recommend using your approach but only logging to the console after/outside the for loop. So it would be called once, after you're done building the string.
var num = ' ';
for ( var i = 4; i <= 156; i += 1 ) {
num += i + ' ' ;
}
console.log(num);