Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial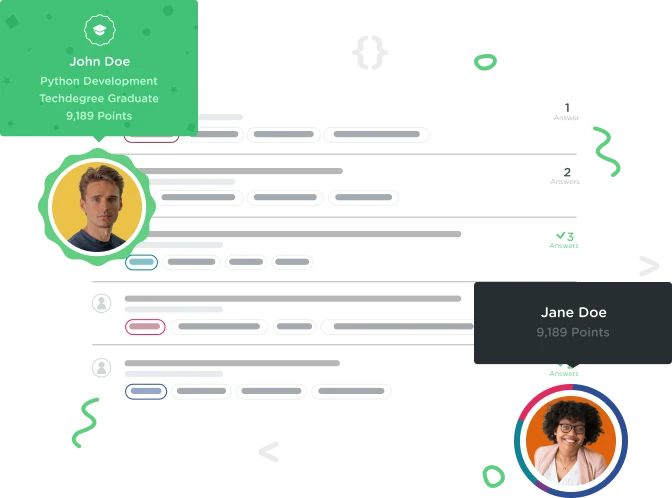
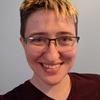
Stephani Dudrak
1,614 PointsI really don't understand 'self'
I'm not getting any useful error, it just says 'bummer, try again' and I don't know what I'm doing wrong.
(For those in charge of course content, please rework this section (self) and probably also the one before it (dicts). The videos do not adequately explain what's happening, and the challenges ask for things the videos didn't cover. I should not be having to ask the community for help on every single challenge, and the fact that I am both slows down my learning and points to insufficiency in the material.)
class Student:
name = "Your Name"
grade = 79
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self.grade):
if grade is > 50:
return praise(self)
elif grade is <= 50:
return reassurance(self)
2 Answers

Brigitte Schuster
23,891 PointsIn the feedback method, you need to call the instance methods praise() or reassurance(), this is done by writing self.praise() and self.reassurance()
Methods are always called with this syntax <instance name>. <class method name>()
Readability note: The ... elif ... statement adds a redundant test, a simple else: does the job.

Brigitte Schuster
23,891 PointsAlmost there! The method feedback takes two arguments self and grade so instead of using a "." you should write feedback(self, grade)
grade is not an attribute of the class Student, it is the second argument of the method feedback()
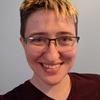
Stephani Dudrak
1,614 PointsThank you for your quick answer! That makes sense, that I'm passing two arguments. Unfortunately I'm still getting a 'bummer, try again' error :(
Stephani Dudrak
1,614 PointsStephani Dudrak
1,614 PointsUpdate: I've rewatched the video at least 3 times and reworked the code in every permutation I can think of. I have no idea why it's not working. (I'm seriously about to quit this course, these videos do not adequately prepare you for the code challenges. Add more chances in the video for the student to try and code, then explain the correct answer. Make the errors actually useful. Add hints after 5 challenge attempts or something. Being stuck on one question for literal hours because the video didn't properly teach me how to code this, and having to wait and rely on community posts is infuriating.)
```class Student: name = "Your Name" grade = 79