Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial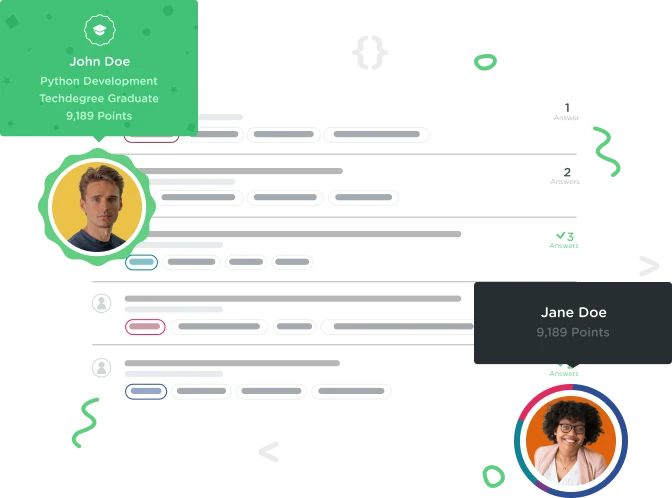

Tojo Alex
Courses Plus Student 13,331 PointsI really don't understand this
Could you give me a brief explanation of what to do.
var birds = new[]
{
new { Name = "Pelican", Color = "White" },
new { Name = "Swan", Color = "White" },
new { Name = "Crow", Color = "Black" }
};
2 Answers

Jared Williams
5,650 PointsThe problem might be because the answer is so simple. All they want you do do is create an anonymously typed variable called "mysteryBird" with the properties "Color" and "Sightings" and fill out the properties. Don't add it to the list, don't use the variable at all, just create the variable. The answer is 1 line of code (if you style it it might take 4, but will only have 1 semicolon). I assume in the next step you will use this "mysteryBird " variable.

Tojo Alex
Courses Plus Student 13,331 PointsI added it though it resulted as this : Did you a new variable with the var keyword named "mysteryBird"?
var new mysteryBird = {Color = "White", Sightings = "3"} ;

Jared Williams
5,650 PointsYou need a new
before the anonymous object you are creating
var myVar = new {Prop1 = "hello", Prop2 = "world"};
The new
keyword indicates to C# to allocate more memory for the new object that is about to be created. You are basically telling C# that you aren't assigning myVar
to an existing object, but that it needs to create a new one in memory and assign it to myVar
.
Also, I believe that Sightings
should be an int, not a string.
Tojo Alex
Courses Plus Student 13,331 PointsTojo Alex
Courses Plus Student 13,331 PointsOh I already figured out what to do (finally) before you posted, though my question now is what is wrong . Error : StudentsCode.cs(13,20): error CS0820: An implicitly typed local variable declarator cannot use an array initializer Compilation failed: 1 error(s), 0 warnings
Code:
Jared Williams
5,650 PointsJared Williams
5,650 PointsYou forgot the "
new
" keyword for your variable.