Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial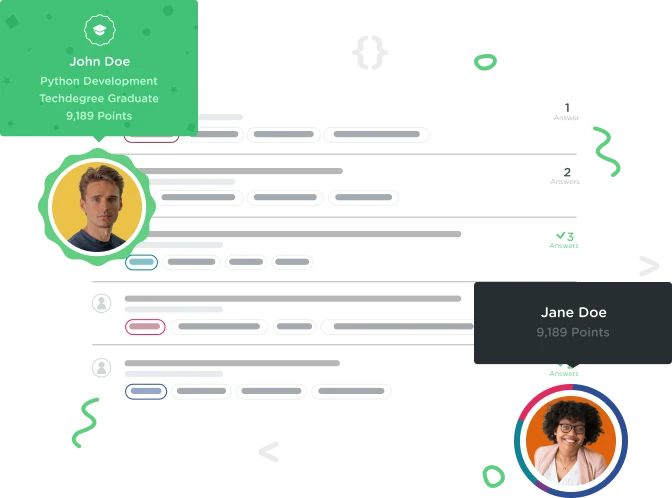

Matthew Deal
5,265 PointsI receive the right answer in playground but cannot even preview my code with errors in challenge
I can return the desired description in play ground but not in the challenge. The challenge return a compiler issue but will not let me see any errors when selecting preview. Below is the code
init() {
red = 86.0
green = 191.0
blue = 131.0
alpha = 1.0
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
var color = RGBColor()
print(color.description)
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init() {
red = 86.0
green = 191.0
blue = 131.0
alpha = 1.0
description = ("red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)")
}
}
var description = RGBColor()
print(description.description)
1 Answer
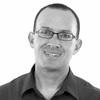
David Papandrew
8,386 PointsHi Matthew,
There are a few things you need to do to get your code to pass:
1) Your custom initializer needs to include parameters for the values you want to assign to your struct stored properties.
2) In the init body, you will set struct stored properties (e.g. self.red, self.blue) to the corresponding values being passed via the init method. See the code below.
3) The RGB values don't go in the init block. Instead, the RGB values are passed in to the init method when an instance of the struct is created.
Here's the code:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = ("red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)")
}
}
//This next part isn't necessary for the challenge, but it shows you how to instantiate the Struct using your custom initializer
//You can mess with this in Playgrounds if you like
let myColor = RGBColor(red: 10.0, green: 100.25, blue: 38.5, alpha: 0.5)
print(myColor.description)
Matthew Deal
5,265 PointsMatthew Deal
5,265 PointsThank you very much for you time and help David. I appreciate you helping beyond the scope of the challenge too. I think I will do another round of these videos to get more familiar with the initializers!