Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial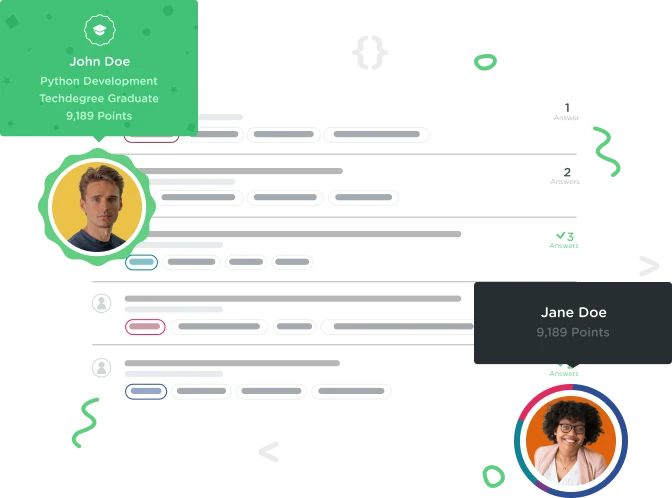

Alex Sabadan
1,654 PointsI received a number, which is greater than the upper number I entered.
This is my code
An inline image
When I input 10 and 100, the program output 2110. What is the problem?
2 Answers
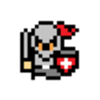
Michael Jurgensen
8,341 PointsHi Alex,
You are passing in a string into your function(prompt returns a number as a string). Instead use parseInt :
// Right after declaring var lower and upper
lower = parseInt(lower,10);
upper = parseInt(upper,10);
lottery(lower, upper);

Alex Sabadan
1,654 PointsThanks Michael!
I think it is the most convenient way to solve the problem.
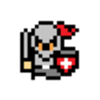
Michael Jurgensen
8,341 PointsNo problem :)

Jason Anello
Courses Plus Student 94,610 PointsHi Alex,
When you get user input with prompt
it's stored as a string.
So you don't have the numbers 10 and 100 but rather the strings '10' and '100'
Your end result is actually the string '2110' and not the number.
This part of your code: upper - lower + 1
ends up working out ok.
You have '100' - '10' + 1
Subtraction isn't overloaded so the operands will be converted to numbers.
You get 100 - 10 + 1
which is what you wanted.
Let's say the random number was 21
after the random and floor operation.
You now have `var roll = 21 + '10';
The +
operator is overloaded. If either argument is a string then the other will be converted to a string and string concatenation will take place.
You end up with '21' + '10"
or '2110'
The result you received.
Hopefully that will help you with how you might troubleshoot your own code in the future.
The easiest fix is to convert that last lower
to a number so that you can avoid the concatenation that takes place in the final step.
You can use the +
unary operator, +lower
var roll = Math.floor(Math.random() * (upper - lower + 1)) + +lower;
As long as your string is already in the form of an integer then the unary plus operator will convert it to a number.

Jason Anello
Courses Plus Student 94,610 PointsI should point out that in real code you would likely be checking that user input to make sure valid numbers were entered and you would likely convert to numbers at that step. So your lottery function would then be getting numbers instead of strings and you wouldn't need to use the unary plus operator.
There's different ways you can handle it.
I think in the next video over you're shown how to use isNaN

Alex Sabadan
1,654 PointsThank you for your answer!
Could you explain something more on the issue. For istance, according to my input, I got '21' and '81', which are both strings. In what manner they are going interact with 1, which is a number istelf?
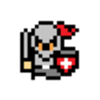
Michael Jurgensen
8,341 PointsI believe the toString() method would be called on the the number one. So the number 1 would actually be " 1". Then it would just concatenate the strings together.

Jason Anello
Courses Plus Student 94,610 PointsAlex,
Could you clarify what you mean there? Which part of the code are you referring to?
And what is the '21' and '81'? Are those what you entered as input?

Alex Sabadan
1,654 PointsAs I've understand, after entering 10 as a lower number and 100 as a upper number I'll get two numbers, for instance "21" and "81", which are actually strings. Am I right here?
Then, they will be added to 1 in this part of the code
var roll = Math.floor(Math.random() * (upper - lower + 1)) + lower;
That's why I'd like to inquire what are rules of addition, substraction and other mathematical operations for two variables, one being a string and second being a number.

Jason Anello
Courses Plus Student 94,610 PointsIf you enter 10 and 100 then you will get '10' and '100' as strings. I'm confused on where the 21 and 81 are coming from.
I'll try to elaborate a little on what I wrote in my answer.
You end up having: var roll = Math.floor(Math.random() * ('100' - '10' + 1)) + '10';
This ('100' - '10' + 1)
is going to be evaluated left to right.
The subtraction will be done first and the operands will be converted to numbers. You can't subtract strings.
You then have this: (100 - 10 + 1)
giving you 91 which is what you want. This part of it works out ok.
The random number that you get from that might be 21 as an example.
Now you have: var roll = 21 + '10';
This is the moment where the code doesn't work right. You'd like to get 31 from this.
Now it's addition and string concatenation will take place giving you '2110'. The number 21 will be converted to the string 21.
As mentioned in my answer, as long as you know you have good input then you can use the unary plus operator to correctly get 31.
var roll = 21 + +'10';
This would give you 31.
And as I mentioned in my follow up comment you likely won't need to do this once you add in code that is properly checking whether you have a valid number or not. The unary plus operator is convenient to use when you know you have integers in the strings.
parseInt() alone isn't enough to check for valid numbers.
The next couple of videos shows how you can use isNaN()
to check if you have a number or not
I hope this helps clear it up for you.
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsI keep coming back to the fact you have a plus + 1 and + lower in your script. I haven't done the course yet but i don';t think you need that.
Try this.
var roll = Math.floor(Math.random() * (upper - lower);