Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial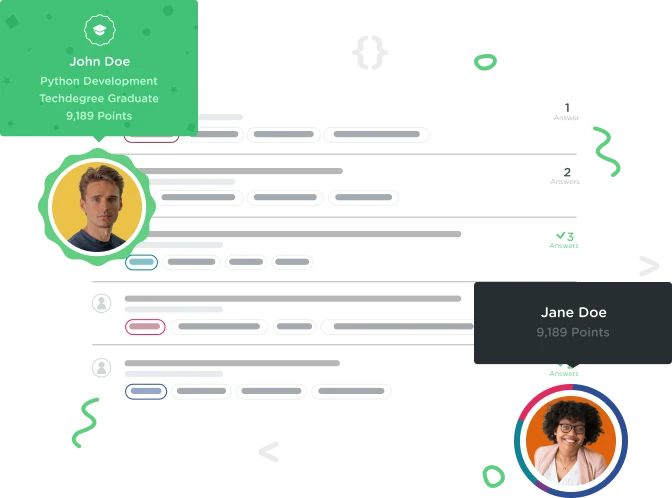

Dare; Rivera
2,297 PointsI recently started coding and I am learning Java, I was wondering if I can get some help with the following program?
The error is at the end, I think is related to my for statement but I do not know what I am doing wrong.
Super class:
public class Ship
{
private String name;
private String year;
public Ship()
{
name=" ";
year=" ";
}
public Ship(String name, String year)
{
this.name=name;
this.year=year;
}
public void setName(String name)
{
this.name=name;
}
public void setYear(String year)
{
this.year=year;
}
public String getName()
{
return name;
}
public String getYear()
{
return year;
}
public String toString()
{
String str="Ship's name is: " + name +
"\nYear it was built was: "+ year;
return str;
}
}
SubCalss:
public CruiseShip()
{
super ();
passangers=0;
}
public CruiseShip(String name, String year, int passangers)
{
super(name, year);
this.passangers=passangers;
}
public void setPassangers()
{this.passangers=passangers;}
public int getPassangers()
{return passangers;}
public String toString()
{
String str= "The ship's name is: " + getName() +
"\nThe ship's capasaity is: "+ passangers;
return str;
}
}
2nd Subclass:
public class CargoShip extends Ship
{
private int cargoCapacity;
public CargoShip()
{
super();
cargoCapacity=0;
}
public CargoShip(String name, String year, int cargoCapacity)
{
super(name, year);
this.cargoCapacity=cargoCapacity;
}
public void setCargoCapacity(int cargoCapacity)
{this.cargoCapacity=cargoCapacity;}
public int getCargoCapacity()
{return cargoCapacity;}
public String toString()
{
String str="Ship's name is: " +getName()+
"\nShip's cargo capacity is: " + cargoCapacity+"tons";
return str;
}
}
Final Demo
public class Fleet
{
public static void main(String[] args)
{
Ship[] group;
group=new Ship[3];
group[0]= new Ship();
group[1]= new CruiseShip();
group[2]= new CargoShip();
group[0].setName("Dungeon");
((Ship)group[0]).setYear("2000");
group[1].setName("Titanic");
((CruiseShip)group[1]).setPassangers(3547);
group[2].setName("Black Pearl");
((CargoShip)group[2]).setCargoCapacity(25000);
for(int i=0; i<group.length; i++)
{
System.out.println(group[i]);
}
}
}```
I get the following error when I compile
Fleet.java:17: error: method setPassangers in class CruiseShip cannot be applied to given types;
((CruiseShip)group[1]).setPassangers(3547);
^
required: no arguments
found: int
reason: actual and formal argument lists differ in length
1 error
2 Answers

Anders Björkland
7,481 PointsHi,
Your setPassengers-method in CruiseShip doesn't take any arguments. I assume you do want a set-method to take an argument, so you probably just missed adding it.
Good luck, and if you have any more questions - continue to ask ^^

Dare; Rivera
2,297 PointsI thought I added the set-method on the CruiseShip subclass, Do i have to add the set-method somewhere else?

Dare; Rivera
2,297 PointsFigured it out thx
Anders Björkland
7,481 PointsAnders Björkland
7,481 PointsHi Rivera,
Could you edit your comment so that the code bits are encapsulated by java markdown? If you edit your post, you see the Markdown Cheatsheet on how to have your code indented and colored in a way to make the code easier to read. That way it is easier for others to help you. Then som java code would look like this: