Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial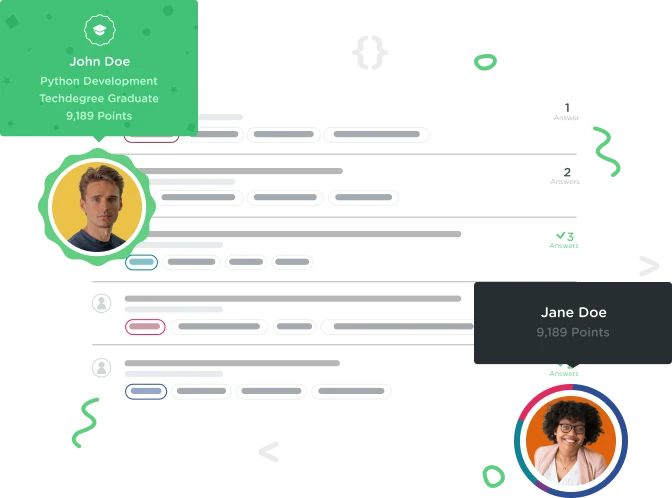

David Bales
3,822 PointsI require assistance.
In the editor, you've been provided with a view controller subclass that contains two instances of UIView as stored properties. The work to set up blueView has already been done; you need to set up greenView.
To set up the green view add the following constraints: Pin to the bottom of blueView with 8 point spacing Pin to left and right margins with 8 point spacing Add height constraint of 75 points.
For this exercise you may use the verbose NSLayoutConstraint APIs or layout anchors to create constraints.
I have 0 ideas on what to do here.
class MyViewController: UIViewController {
let blueView = UIView()
let greenView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(greenView)
view.addSubview(blueView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
blueView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activateConstraints([
NSLayoutConstraint(item: blueView, attribute: .Height, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 100.0),
NSLayoutConstraint(item: blueView, attribute: .Width, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 150.0),
NSLayoutConstraint(item: blueView, attribute: .Left, relatedBy: .Equal, toItem: view, attribute: .LeftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .Top, relatedBy: .Equal, toItem: self.topLayoutGuide, attribute: .Bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .Right, relatedBy: .Equal, toItem: view, attribute: .RightMargin, multiplier: 1.0, constant: -8.0)
])
// Add constraint code below
}
}
2 Answers

U Belfast
6,439 PointsHi,
Well, you can find the hint which add height constraint of 75 points to the greenView below: It is left to add another 3 constraints
class MyViewController: UIViewController {
let blueView = UIView()
let greenView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(greenView)
view.addSubview(blueView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
blueView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activateConstraints([
NSLayoutConstraint(item: blueView, attribute: .Height, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 100.0),
NSLayoutConstraint(item: blueView, attribute: .Width, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 150.0),
NSLayoutConstraint(item: blueView, attribute: .Left, relatedBy: .Equal, toItem: view, attribute: .LeftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .Top, relatedBy: .Equal, toItem: self.topLayoutGuide, attribute: .Bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .Right, relatedBy: .Equal, toItem: view, attribute: .RightMargin, multiplier: 1.0, constant: -8.0)
])
// Add constraint code below
NSLayoutConstraint.activateConstraints([
NSLayoutConstraint(item: greenView, attribute: .Height, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 75.0),
])
}
}
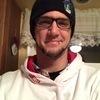
Ryan Sady
20,594 PointsHi David,
I'm not sure if you've figured out the issue or not, but you need to use upper camel case for the attributes. .Height, .Width, .NotAnAttribue (which was illustrated above by U Belfast).