Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial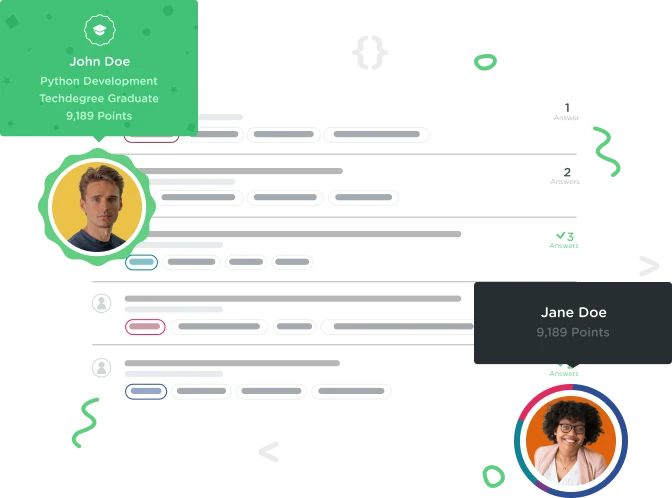

Hudhayfee Hassan
1,787 PointsI seem to be getting the same Error message when running the Binary Search Algorithm myself...not sure why?
def binary_search(list, target):
first = 0
last = len(list) - 1
while first <= last:
midpoint = (first + last)//2
if list[midpoint] == target:
return midpoint
elif list[midpoint] < target:
first = midpoint + 1
else:
last = midpoint - 1
return None
def verify(index):
if index is not None:
print("Target found at index: ", index)
else:
print("Target not found in list")
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = binary_search(numbers, 12)
verify(result)
result = binary_search(numbers, 6)
verify(result)
MOD: I updated your code so that it's formatted, and others can better help you. You can find out how to do this in the Markdown Cheatsheet that is right below the textbox when you're entering a question. Thanks!

Hudhayfee Hassan
1,787 Pointsthe line that reads...
return None
...is where the error is.
3 Answers
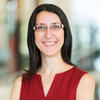
Louise St. Germain
19,425 PointsHi! With the formatting in place it's much more obvious - everything from while first <= last:
all the way to (and including) return None
needs to be indented one more level. Otherwise, none of that is inside the binary_search method you defined.
Give it a try and see if it works!
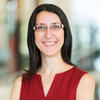
Louise St. Germain
19,425 PointsActually that if statement needs to be indented 2 more levels so that it's inside the while loop. Otherwise, you're not modifying first or last and it will cycle endlessly in the while loop...

Hudhayfee Hassan
1,787 PointsIt works!!!
Thanks for your help.
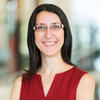
Louise St. Germain
19,425 PointsYou're welcome! Glad you got it working.

Hudhayfee Hassan
1,787 PointsI've tried to indent...no success I'm afraid

Hudhayfee Hassan
1,787 PointsIt's now showing a syntax Error on that same line
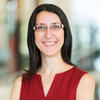
Louise St. Germain
19,425 PointsHi!
It's working for me with no change other than the indenting. Make sure it looks like this:
def binary_search(list, target):
first = 0
last = len(list) - 1
# This entire while loop is indented one level so it's inside binary_search
while first <= last:
midpoint = (first + last)//2
# This entire if statement is indented 2 levels so it's inside the while loop
if list[midpoint] == target:
return midpoint
elif list[midpoint] < target:
first = midpoint + 1
else:
last = midpoint - 1
# The return statement is indented 1 level. It's inside the while loop, but not the if statement
return None
def verify(index):
if index is not None:
print("Target found at index: ", index)
else:
print("Target not found in list")
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = binary_search(numbers, 12)
verify(result)
result = binary_search(numbers, 6)
verify(result)
Also, double-check that you don't have a mix of spaces and tabs when you indent, because that will cause a problem.
If that still doesn't work, please let me know what syntax error you're getting.
KRIS NIKOLAISEN
54,972 PointsKRIS NIKOLAISEN
54,972 PointsOther than the formatting your code appears to match the code in the video. What error are you getting?