Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial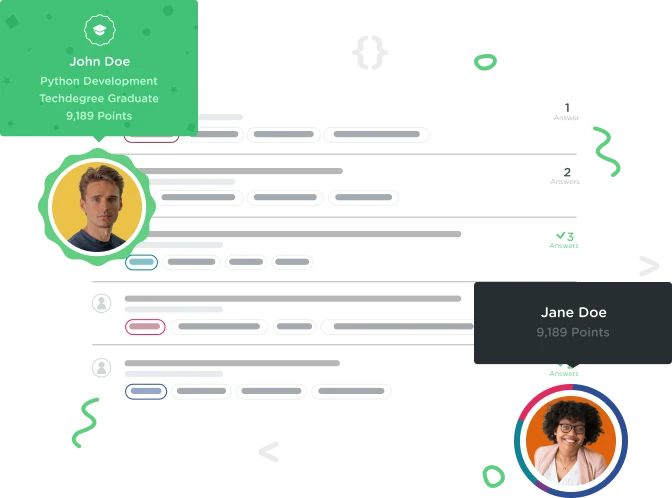

Faris Zaman
2,802 PointsI seem to keep getting an error saying "Variable used within its own initial value" on this task.
I feel the problem lies in the fact the function is named "greeting" and a constant also within the function is named greeting.
e.g.
func greeting(#person: String) -> (language: String, greeting: String) { let language = "English" let greeting = "Hello (person)" return (language, greeting) }
Thus when trying to call upon a final result, it would end up looking like this?
let (language: String, greeting: String) = greeting(person: "Tom")
let result = greeting(person: "Tom")
result
However, it highlights the repetition of greeting twice in the code where I am trying to draw a result, I've tried to rename the function from the constant yet the complier seeks an answer in the form of greeting. Can you please highlight where my flaw in understanding this concept is please? Thank you.
func greeting(#person: String) -> (language: String, greeting: String) {
let language: String = "English"
let greeting: String = "Hello \(person)"
return (language, greeting)
}
let (language: String, greeting: String) = greeting(person: "Tom")
let result = greeting(person: "Tom")
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsThe problem is the following line of code:
let (language: String, greeting: String) = greeting(person: "Tom")
You don't specify a name for the constant here, which is mandatory,
in this case you are asked to name it result
:
let result: (language: String, greeting: String) = greeting(person: "Tom")
No need to do that extra step in between, you can assign a tuple to a variable right away.
You can even omit the type annotation (language: String, greeting: String)
as Swift can infer those, it knows that the function greeting
returns a tuple and what it contains. So your code would look like this:
func greeting(#person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
var result = greeting(person: "Tom")
Hope that helps :)
P.S.
Please note that the order of the tuple to return has to be 'greeting' first and 'language' second as described in the assignment. You'll get away with it at the first part of the assignment, but it will be an error with the next steps.
Faris Zaman
2,802 PointsFaris Zaman
2,802 PointsThank you ever so much Martin :)!! Really cleared things up, and taught me a few new short cuts! I can't believe I didn't name the constant >.<!