Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial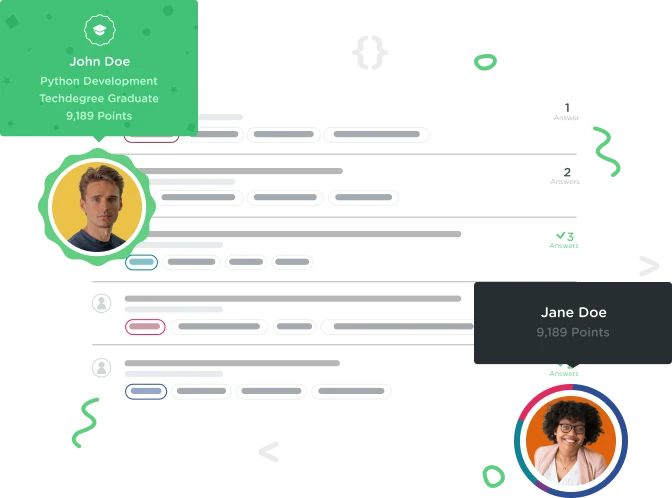
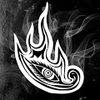
Ian Ross
12,910 PointsI Simply Don't Understand the Instructions: How Does the Last Video Help with This?
I've tried every which way I can think of to get this code challenge to work and move on, and the compiler errors have been pretty useless (I know they're for technicalities anyway). My question is unfortunately rather broad (I do regret how general this is, but I'm stuck!): What is it about the last video's For Each Loop explanation that is applicable to this new coding challenge?
I apologize again, sincerely, for the broad question, but I cannot for the life of me figure out how to solve this based on what was recently taught, since that is where the info for the previous challenges was presented. I want to move on and continue with the lessons but I'm stumped...
Sorry again.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
}
2 Answers
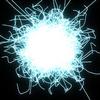
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Ian,
This challenge is kind of a rough introduction to how things change in the Java Track. It's not as straight forward as the other questions by design. Moving forward a lot of challenges are going to require that you think a little more about them, which is good, as it truly helps you understand the concepts that you apply to solve these problems.
So let's break it down, step by step.
1.) we need an array that is obviously supposed to return an int, so we should use that in the method signatures, also, let's pass in the char that we want to check and see if it's contained in our hand.
public int getTileCount(char tile) {
}
Simple enough.
Now, we want this method to reset it'self each time we use it, so let's set an int value at the top that is set to zero.
public int getTileCount(char tile) {
int count = 0;
}
So far so good.
Now we've reached a problem. We can use the getHand() to get our hand.. as a String value, which does us very little good for the context of what we find out. Thankfully. toCharArray()
Does this job quite nicely.
Okay, let's set up our loop, remember that we're looping over each char in the array and checking it if it matches the char passed in as an argument and increment our count by one if the values match. Something like below
public int getTileCount(char tile) {
int count = 0;
for (char checkedTile : getHand().toCharArray()) {
if (checkedTile == tile) {
count++;
}
}
}
That's simple enough, and the final touches are going to be return our count variable and hope that the method has been incrementing it as hoped. it would look below
public int getTileCount(char tile) {
int count = 0;
for (char checkedTile : getHand().toCharArray()) {
if (checkedTile == tile) {
count++;
}
}
return count;
}
Thanks hope this helps!
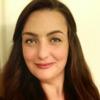
Jennifer Nordell
Treehouse TeacherI can give you some hints here. Craig talks about something very similar to this challenge at about 1:52 in the video.
But let's think for a second about what it's actually asking you to do. We have a Scrabble hand, right? Now if you've ever played Scrabble (and I'm guessing you have), you know that at any given time you may have one or more of a given letter in your hand. You could have for example four "z"s in your hand. And good luck winning that game!
So we've already make a function to see if a given letter exists in our hand. Do we have a z? Yes, in fact, we have 4. But because this only returns a true or false we only know that we have "z"... not how many.
We're writing a new function to determine how many of those silly "z"s we have. This function accepts one char
named tile
. We're going to convert the hand to a character array and go down that list. This is where the for
loop is going to come in handy Every time we hit the letter 'z' we increment our count. Note that you will have to create a variable to hold the total number of "z"s that you find.
When we get to the end we return the number of "z"s in our hand. I hope this helps!