Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial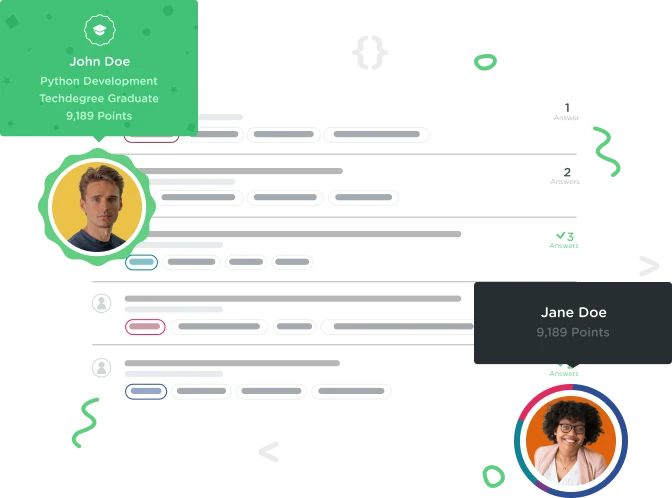
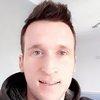
Russell Smitheram
2,009 PointsI solved the challenge but did I do it a long winded way?
I gave this challenge a shot and solved it but I feel I've done it a really long winded way.
Can anyone have a look and give me some feedback please?
Workspace snapshot - https://w.trhou.se/ypgwqlrl3k
I added the console.log() to the beginning and end to help with debugging as I went.
6 Answers
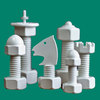
Steven Parker
231,275 PointsYour program seems quite compact as it is. You could split up the assignment of "message" into several smaller statements to have shorter lines (but more of them). Or you could employ functions to change the program structure,. But neither of these would significantly condense this program.
These would be good techniques to apply to larger programs, and you'll see examples of both as you continue with the courses.
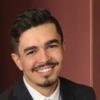
kaganulucay
Full Stack JavaScript Techdegree Student 16,491 PointsHere is the more compact way just take a look
alert(`Let's do some math!`);
const number1 = prompt('Please type a number');
const number2 = prompt('Please type another number');
document.writeln(`
<h1>Math with the numbers ${number1} and ${number2}</h1><br>
${number1} + ${number2} = ${number1 + number2}<br>
${number1} * ${number2} = ${number1 * number2}<br>
${number1} / ${number2} = ${number1 / number2}<br>
${number1} - ${number2} = ${number1 - number2}<br>
`)
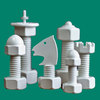
Steven Parker
231,275 PointsCompact, yes, but without the numeric conversions, the "+" operator performs concatenation on the two strings.
"2 + 3 = 23
"
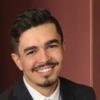
kaganulucay
Full Stack JavaScript Techdegree Student 16,491 PointsOkay so here you go:
alert(`Let's do some math!`);
const number1 = prompt('Please type a number');
const number2 = prompt('Please type another number');
calculation = (number1,number2) => {
document.writeln(`
<h1>Math with the numbers ${number1} and ${number2}</h1><br>
${number1} + ${number2} = ${number1 + number2}<br>
${number1} * ${number2} = ${number1 * number2}<br>
${number1} / ${number2} = ${number1 / number2}<br>
${number1} - ${number2} = ${number1 - number2}<br>
`)
}
if(number1 == 0 || number2 == 0){
alert(`You can't divide by zero. Reload and try again.`);
}
else if(isNaN(number1) == true || isNaN(number2) == true){
alert(`At least one of the values you typed is not a number. Reload and try again.`)
}
else{
calculation(number1,number2);
}
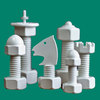
Steven Parker
231,275 PointsI see you added some error checking, but the situation I was pointing out occurs with normal inputs. For example, if the numbers 2 and 3 are entered, you'll get: "2 + 3 = 23
". The revised code performs the same in that regard.
But in itself, sanity checking on user inputs is always a good idea for deployed code.
And I posted a comment to my original answer with an example using a function.
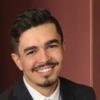
kaganulucay
Full Stack JavaScript Techdegree Student 16,491 PointsOh so I would probably use Number() function
${number1} + ${number2} = ${Number(number1) + Number(number2)}
Something like this. Or I would apply that when I prompt the number.
const number1 = Number(prompt('Please type a number'));
const number2 = Number(prompt('Please type another number'));
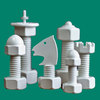
Steven Parker
231,275 PointsThere ya go. Either that or "parseFloat" as Russell had and you're good.
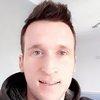
Russell Smitheram
2,009 PointsThank you for your reply, Steven. Much appreciated.

Curtis Chadwell
3,150 PointsI did it the same way to help me read it better.
alert('Math Time!'); var firstNum = prompt('What is your first number?'); firstNum = parseFloat(firstNum); var secondNum = prompt('What is your second number?'); secondNum = parseFloat(secondNum); var addNums = firstNum + secondNum; var subNums = firstNum - secondNum; var mulNums = firstNum * secondNum; var divNums = firstNum / secondNum;
var message = '<h1>Math with the numbers ' + firstNum + ' and ' + secondNum + '</h1><br>'; message += firstNum + ' + ' + secondNum +' = ' + addNums; message += '<br>'; message += firstNum + ' * ' + secondNum + ' = ' + mulNums; message += '<br>'; message += firstNum + ' / ' + secondNum + ' = ' + divNums; message += '<br>'; message += firstNum + ' - ' + secondNum +' = ' + subNums;
document.write(message);
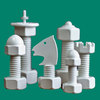
Steven Parker
231,275 PointsTo make your code look better, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
 Or watch this video on code formatting.
You could also make a snapshot of your workspace and post the link to it here, as Russell did.

Curtis Chadwell
3,150 PointsI copy and pasted from a different editor, and it looked like that after I submitted it. It was clear before.
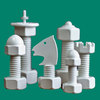
Steven Parker
231,275 PointsSure, it changes when you post it in the forum because the forum is processing the posts with "Markdown".

Curtis Chadwell
3,150 PointsI see, thanks
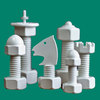
Steven Parker
231,275 PointsTry using one of my suggestions to edit your code above to make it appear correctly.
Dane Parchment
Treehouse Moderator 11,077 PointsDane Parchment
Treehouse Moderator 11,077 PointsGreat answer Steven, you beat me to it.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsSince there seems to be continued interest, here's an example implementing my function suggestion: