Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial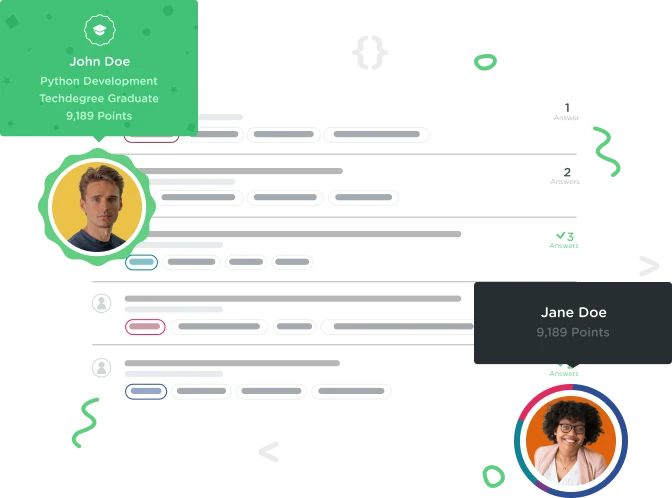

Rachel Ny
3,073 PointsI still really don't understand what an initializer does. please help me.
I don't understand how to create an initializer for this class because I'm still confused as to what an initializer does. Can someone help me understand? Maybe through some metaphor or something?
// Enter your code below
class Shape {
var numberOfSides: Int
init () {
self.numberOfSides = numberOfSides
}
}
let someShape = Shape()
3 Answers

Josh Kuehn
8,227 PointsGreat question!
Initializers are functions you create in a class that allow you to create an instance of an object in one or more ways. Without them you can't create an instance of that class.
Imagine someone is building you a home. This builder brings you a list of items he needs to complete the construction of your home. If you bring him all these items he'll be able to build you a nice home. In the same way when you fill out an initializer you're providing your class the things it needs to create an instance of that class.
Now imagine the same home above but someone else started building it and then abandoned it. Now you hire a builder to complete the home but this time you don't need all of the items as above because it's already halfway built. That's similar to creating another initializer that can create the same object but requires more or less variables to create an instance of a class.
Take a look at the code below to see an example of a Person class with 3 different initializers being used.
//: Playground - noun: a place where people can play
import UIKit
class Person {
//Person attributes initialized to blank values to avoid nil
var name = ""
var age = ""
var favoriteColor = ""
//Default initializer
init() {
name = "John Smith"
age = "24"
favoriteColor = "Red"
}
//Initializer with name and age options
init(name: String, age: String) {
self.name = name
self.age = age
favoriteColor = "Red"
}
//Initializer with name, age, and favoriteColor options
init(name: String, age: String, favoriteColor: String) {
self.name = name
self.age = age
self.favoriteColor = favoriteColor
}
}
//Default Initializer
var stranger = Person()
//Initializer with name and age options
var neighbor = Person(name: "Helga Ortiz", age: "33")
//Initializer with name, age, and favoriteColor options
var bestFriend = Person(name: "Seth Banks", age: "27", favoriteColor: "Purple")
The above example illustrates how one class can have multiple initializers. You can see each one gives me different options to create the class. This is useful because I don't always want to initialize every variable of a class and other times I want to be very specific.
I hope this helps. Initializers were one of the more complicated parts of Swift when it was introduced so it's not uncommon to feel confused about this. Just remember StackOverflow is your best friend as a developer :)

andren
28,558 PointsA common metaphor for classes in general is that they are like blueprints and instances of them are like objects built from said blueprint. In that metaphor the initializer would be like the person who is actually responsible for taking the blueprint and building an object out of it.
In less metaphorical terms an initializer is a method you call which returns an instance of the class. It is responsible for setting the class up, so if you have some variables that need to be set to some value when the class is created that comes from outside the class then that is the method where that assignment needs to happen.
When you call Shape()
you are actually calling the init
method. of the Shape
class. Your code is actually pretty close to correct, but you need to change the init
method to actually take a numberOfSides
parameter (currently it takes none), and then pass in an Int
when you instantiate the class.

Rachel Ny
3,073 PointsThank you Andren and John. I found this very helpful! I especially really liked the house metaphor. Really appreciate.