Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial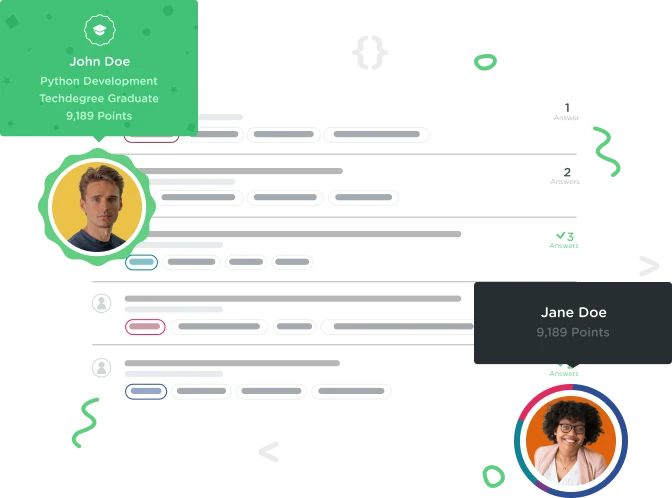

Aaron Arkens
2,343 PointsI think I did the FizzBuzz challenge correctly but it's not letting me pass?
I can't tell if there's an error with my code or with the site. It seems to check out fine in xcode.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
for n in 1...100 {
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
} else if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
} else {
return n
}
}
// End code
return "\(n)"
}
3 Answers
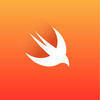
Steven Deutsch
21,046 PointsHey Aaron Arkens,
Your FizzBuzz solution is a working one. You're just not formatting it how the challenge is asking so that it can check your answer. You need to remove the last else clause and your loop.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
} else if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
}
// End code
return "\(n)"
}
Good Luck!
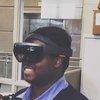
Guled Ahmed
12,806 PointsHello Aaron!
There is no reason to use a loop within your function (this would work if you were given an array of numbers to evaluate). Your function simply needs to take in the input and then evaluate what the user had passed into the function. When you remove your loop your code should work.
Example ( I fixed up some of the code)
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz"
} else if (n % 3 == 0) {
return "Fizz"
} else if (n % 5 == 0) {
return "Buzz"
} else {
return String(n)
}
return ""
}
With this example, I'm directly working with n, the users input. The code you posted does not compile correctly. Your function is suppose to return a String type, but at the end of your if-else clause you did:
return n
Which would throw an error. If you want that to be a string, use the String function:
return String(n)
Hope this helped. :)

Aaron Arkens
2,343 PointsThanks guys! I'll try it out.