Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial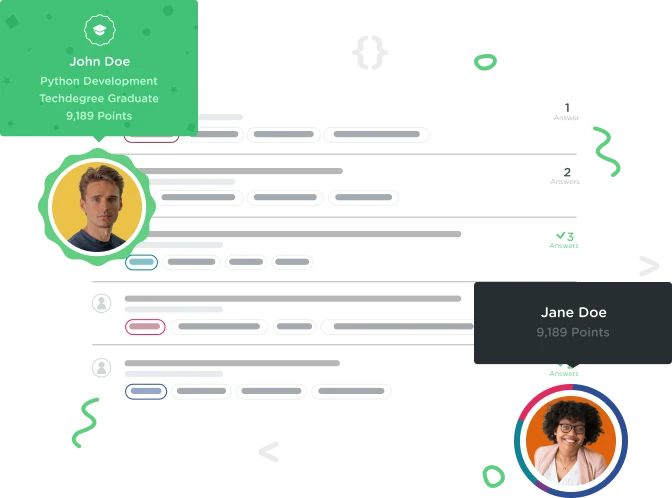

Jason Smith
8,668 PointsI think i have all the functions i need, what is wrong?
i'm not sure where i made the error
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(sentence):
sentence.lower()
word_list = sentence.split()
dic = {}
number = 1
for word in word_list:
number = word.count
dic = dic.format{},{}(word_list, number)
2 Answers
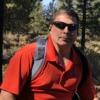
Mark Kastelic
8,147 PointsJason,
Starting with your code, I made a few modifications to make it work.
- Since strings are immutable, sentence.lower() will not save the lower case version unless you assign it to a variable (just reassign as in line 2).
- No need to initialize "number" to 1 since it is not being used as a counter, but rather is assigned the result of the built-in count method run on the word_list with the search parameter being each word.
- As mentioned in 2 above, the count method takes 1 parameter, which is the string it's looking for. In this case we need to search the entire word_list, and as you correctly looped through the individual words of this list with "word", that is the string we want count to look for each time in the word_list.
- The "format" method works on strings, not dictionaries. To build the dictionary you only need assign each key (word) in the dictionary to it's word count (note: since the for loop comes across "I" twice, dic["I"] = 2 is executed twice, making this way of doing things a little redundant, but still correctly getting the job done).
Finally, I believe most of the challenges want to "see" a return statement at the end. Just for testing, I added a print statement to the script.
def word_count(sentence):
sentence = sentence.lower()
word_list = sentence.split()
dic = {}
for word in word_list:
number = word_list.count(word)
dic[word] = number
return dic
print(word_count("I do not like it, Sam I am"))
Now, if you study the collections standard library, you'll find a handy function called "Counter" and can simplify even further as below:
from collections import Counter
def word_count(sentence):
sentence = sentence.lower()
word_list = sentence.split()
return Counter(word_list)
Try it!

Jason Smith
8,668 PointsThis helps alot, i'll take a second swing at it, Thanks!