Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial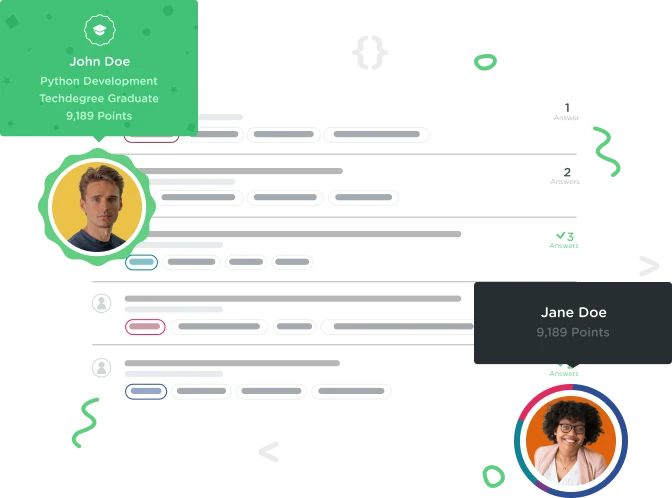
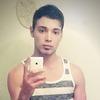
Ryan Burgess
593 PointsI think I understand what a prototype is.
Pretty much a snippet of a property that belongs to an object literal. Instead of creating an object literal with every property needed. You use prototype to create a snippet of it so when you create an instance that doesnβt use that certain property it won't run it to save RAM. Instead, you can chain to other instances that use that property?
2 Answers
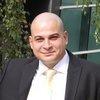
Tsenko Aleksiev
3,819 PointsOk, as far as I know it the prototype is also an object and all javascript objects inherit their properties and methods from their prototype. Think of it like this: you have a car factory and you must have a prototype that is set in the computer and you just click how many cars do you want produced and just set different properties for them aka:
function Car(color, engine, power){
this.carColor = color;
this.engineType = engine;
this.carPower = power;
}
After that you set each cars properties:
var myCar = new Car("black", "diesel", 250);
I think that's it :)
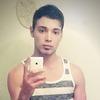
Ryan Burgess
593 PointsI did some more research and this is what I got. prototypes let you encapsulate code and share the code with related objects very easily. If you have a function that generates a random number inside the constructor function. Every new instance you create will create their own random number generator. Instead of having every new instance creating their own random number function. You use prototype so It would instead look for the function in the prototype chain and in its parents when you call it.
function Dice(sides) {
this.sides = sides;
}
Dice.prototype.roll = function () {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
var dice = new Dice(6);
var dice10 = new Dice(10);
console.log(dice.roll());
console.log(dice10.roll());
Is that right?