Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial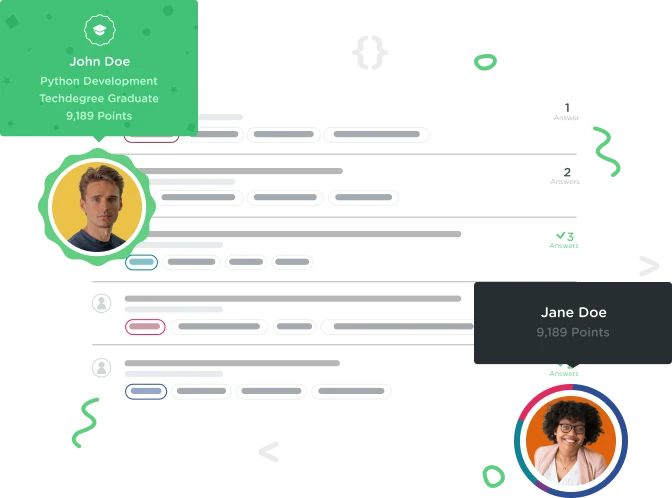
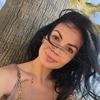
Iulia Maria Lungu
16,935 PointsI think the for loop should be done differently. Incrementing on each iteration sounds weird to me even if it's working.
Keeping an increment in the loop is weird and not relying on the list index. I was expecting something different since this is a tutorial. So this is more like a feedback from my side.
# TODO Create an empty list to maintain the player names
team = []
# TODO Ask the user if they'd like to add players to the list.
answer = input('Want to add players to the list? yes/no \n')
# If the user answers "Yes", let them type in a name and add it to the list.
while answer.lower() == 'yes':
player = input('Tell me the player name please \n')
if player:
team.append(player)
answer = input('Want to add MORE players to the list? yes/no \n')
else:
print('Please type a name. I got an empty name')
# If the user answers "No", print out the team 'roster'
print("The team is: {}".format(team))
# TODO print the number of players on the team
team_size = len(team)
print("The team has {} players".format(team_size))
# TODO Print the player number and the player name
# The player number should start at the number one
for index in range(team_size):
print('Player {}: {}'.format(index + 1, team[index]))
# or
for key, val in enumerate(team, start=1):
print('Player {}: {}'.format(key, val))
# TODO Select a goalkeeper from the above roster
# TODO Print the goal keeper's name
# Remember that lists use a zero based index
try:
goalkeeper = team[1]
except IndexError as err:
print('No goalkeeper set. There are only {} members in the team'.format(team_size))
else:
print('The goalkeeper will be {}'.format(goalkeeper))
This is my solution after searching on how to loop and make use of indexes in Python. Feel free to comment! Thanks
1 Answer
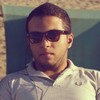
Noor Elharty
2,630 PointsThat seems like a good approach , even i don't understand some of the keywords in it , like key or var ... yet. Ken's approach (incremting and not rely on indexing) seems to me like iq testing more than coding. I was banging my head to wall for an hour trying to figure it out ... Untill i come up with this solution (somehow relying on indexing )
for player in player_names:
player_number = player_names.index(player) + 1
print(player_number, player)
It's still weird solution but that's the best i could find with my current knowledge. Your solution seems more professional not work-aroundish like mine , so if you have references or links to help me understand your approach , it would be great
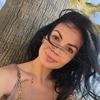
Iulia Maria Lungu
16,935 PointsThis reference here is really good, @Noor Elharty !
Basically for me key
is the index
and val
(value) is the player name
Muhammad khan
Python Development Techdegree Student 1,836 PointsMuhammad khan
Python Development Techdegree Student 1,836 PointsHello, how are you asking the user for goalkeeper selection? It seems player 2 in the list will be selected as the goal keepers? team[1]