Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial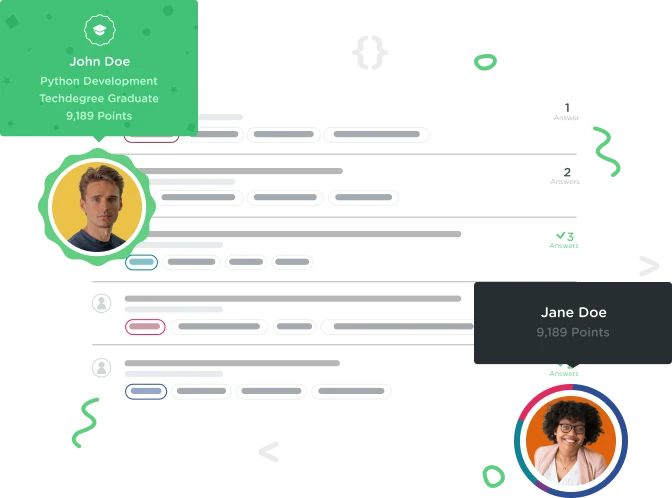

Lee Wise
1,335 PointsI think this challenge is broken
Getting this error: Frog.cs(3,16): error CS1644: Feature `primary constructor' cannot be used because it is not part of the C# 6.0 language specification Compilation failed: 1 error(s), 0 warnings
namespace Treehouse.CodeChallenges
{
class Frog ()
{
public readonly int TongueLength;
Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
2 Answers

andren
28,558 PointsThe challenge is fine, there are two errors in your code:
You have parenthesis after "Class Frog" which should not be there, class declarations does not use parenthesis.
You have not used an access modifier like public or private when declaring the constructor, which you need to do. Specifically for this case you need to make it public.
Fixing those two errors, like this:
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
Will allow you to complete the challenge.

Cheikh Faye Ndiaye
7,298 Pointsthank you andren, you help me.
Patrick McLeod
5,913 PointsPatrick McLeod
5,913 PointsHi I was stuck on the same question and eventually gave up and found the answer here. Still donΒ΄t understand why I was supposed to state Frog as public though, If you could expand on the answer I would appreciate it. Cheers, PM
andren
28,558 Pointsandren
28,558 PointsSure Patrick McLeod I can expand on my answer.
If you don't include an access modifier (
public
,private
, etc) when you declare something in a class then C# will set its access level toprivate
by default. When the access level isprivate
you can only access that thing within the class where it was declared.The purpose of a constructor is to create an instance of the class, or put more simply to create an object. It is called automatically when you create an object. So this line of code for example:
Frog frog = new Frog();
Would end up calling the
Frog
constructor. However if a constructor isprivate
then it can only be called within the class. Which means that you would be unable to createFrog
objects anywhere besides inside of theFrog
class itself.One of the main points of classes is that you can create instances of them within other classes, so having a
private
constructor is extremely rare.public
things on the other hand can be accessed from any class in your program, and are therefore what most constructors are declared as.