Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial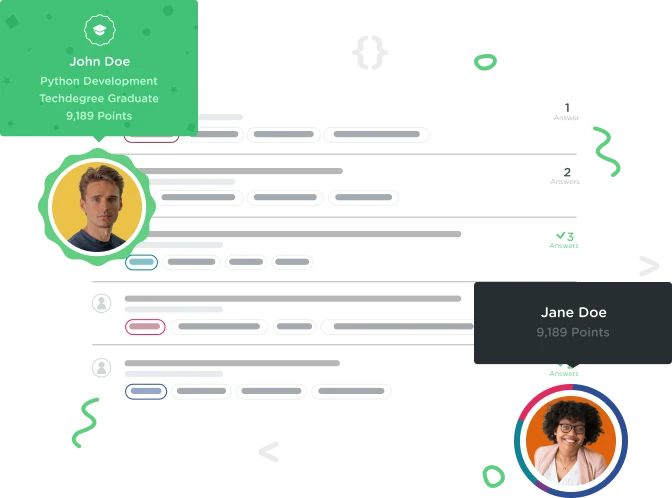
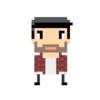
Scott Wells
8,715 PointsI though .reduce(); made a new array, not a new object?
In a previous video, he taught us that ".reduce();" uses two parameters, with the initial accumulator value defaulting to the first element in the array...
users.reduce((usersObject, user) => {});
...but he adds curly brackets to his code, and I'm not sure what it does.
const newUsersObject = users.reduce((usersObject, user) => {
usersObject[user.name] = user.age;
}, {});
Does this create a new object? If so, how? I thought it just referenced the array?
And also, I'm not sure what's happening in the callback function. Why is "usersObject" next to "user.name" in brackets?
1 Answer
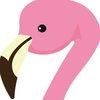
Dave StSomeWhere
19,870 PointsHey Scott, best first place to start is with the MDN reduce documentation.
Please refer to the docs for the available (mandatory and optional parameters) - hopefully that and the description below will answer your question. It also provides a good walkthrough of what happens inside the reduce().
Let's look into why the curly braces at the end and the object/brackets question...
Here's basically the same code with some different names and also including the needed return. Hopefully the comments explain what you are asking. Just let me know if more detail is needed.
Hope this makes sense:
const users = [
{name: 'Samir', age: 22},
{name: 'Angela', age: 32},
{name: 'Beatrice', age: 42}
];
/*
create a new variable called usersObject
groupOfUsers is the accumulator
groupOfUsers is initialized as an object (curly braces at end)
userInstance is the individual entry in the users array that is looped through
groupOfUsers[userInstance.name] is creating a new object key for each name
userInstance.age is the value being assigned to the new object key each time
return groupOfUsers puts each object entry (each time through the loop) into usersObject
*/
const usersObject = users.reduce((groupOfUsers, userInstance) => {
groupOfUsers[userInstance.name] = userInstance.age;
return groupOfUsers
}, {}); // the curly braces is the initial value of an empty object
console.log(usersObject); // show what we created in the console
// console output
{ Samir: 22, Angela: 32, Beatrice: 42 }
Chris Adams
Front End Web Development Techdegree Graduate 29,422 PointsChris Adams
Front End Web Development Techdegree Graduate 29,422 PointsThanks Dave, this explanation helped a lot!