Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial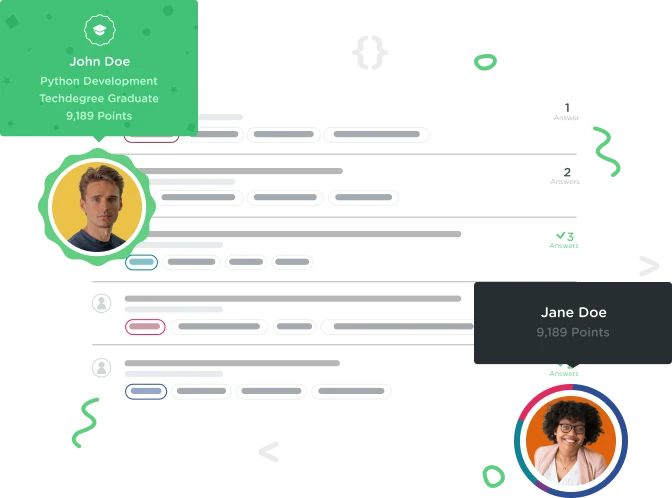

Soheb Vahora
9,229 PointsI thought const variables were immutable, how do we use const for this and are able to change the name property?
We are taught to use 'let' or 'var' to define variables that we will mutate, but 'const' should be used for variables that we don't plan on changing. In this example 'const' is used for the object and then we proceed to change the name property within the object. What benefit does 'const' provide if it is mutable in this instance?
https://teamtreehouse.com/library/set-the-value-of-object-properties
1 Answer

ygh5254e69hy5h545uj56592yh5j94595682hy95
7,934 PointsI'll try to explain it to the best of my abilities.
// The following line will create an object called person
const person;
// This object is now create and cannot be modified because of the const
// if you do this you will get an error because the person object has been already create and is immutable
person = 'me';
// This is the right way to do this
const person = 'me';
// Now you have an object that is immutable and is referring 'me'.
// As for the video that you are referring to:
const person = {
name: 'Edward',
city: 'New York',
age: 37,
isStudent: true,
skills: ['Javascript', 'HTML', 'CSS']
};
// in this case the object person has been create and the object itself is immutable,
// but this object also has properties like, name, city, age, isStudent, skills.
// Those properties are mutable, you can change the properties but not the object itself.
// Here is how to change the properties
person.name = 'Steve';
person.city = 'London';
// You can also add more properties to the object
person.position = 'Lead Programmer';
person.description = 'I like to code';
// Here is what you can't do because the person object has already been declared above
const person = 'me';
const person = {
name: 'Mike',
age: 20
};
Hopefully, this help!