Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial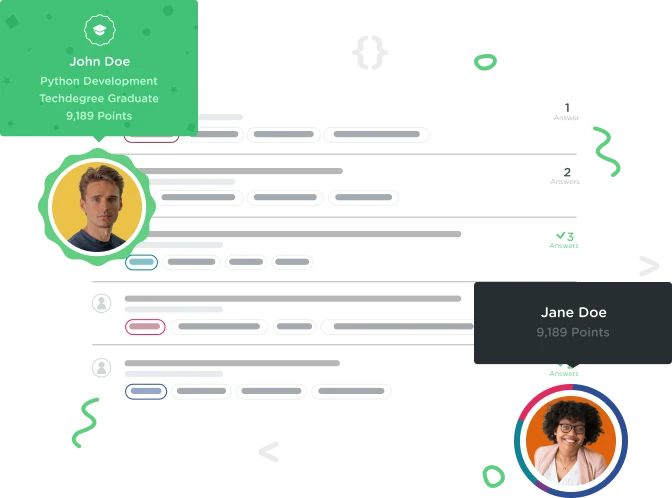
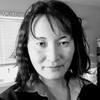
Sarah A. Morrigan
14,329 Pointsi thought of doing something to make it more interesting
#!/usr/bin/ruby
class Monster
attr_reader :name, :actions
def initialize(name)
@name = name
@actions = {
screams: 0,
scares: 0,
run: 0,
hide: 0
}
end
def say(&block)
print "#{name} says "
yield
end
def scream(&block)
actions[:screams] += 1
print "#{name} screams "
yield
end
def scares(&block)
actions[:scares] += 1
print "#{name} scares "
yield
end
def run(&block)
actions[:run] += 1
print "#{name} runs "
yield
end
def hide(&block)
actions[:hide] += 1
print "#{name} hides "
yield
end
def print_scoreboard
puts "-" * 25
puts "#{name} scoreboard"
puts "-" * 25
puts "- Screams: #{actions[:screams]}"
puts "-" * 25
puts "- Scares: #{actions[:scares]}"
puts "-" * 25
puts "- Runs: #{actions[:run]}"
puts "-" * 25
puts "- Hides: #{actions[:hide]}"
end
end
monster = Monster.new("Fluffy")
xsay = rand(1..9)
xsay.times do
monster.say do
puts "welcome to my home"
end
end
xscream = rand(1..100)
xscream.times do
monster.scream do
puts "BOO!"
end
end
xscares = rand(1..100)
xscares.times do
monster.scares do
puts "Go away!"
end
end
xrun = rand(1..100)
xrun.times do
monster.run do
puts "Going to get you!"
end
end
xhide = rand(1..100)
xhide.times do
monster.hide do
puts "running away and hiding!"
end
end
puts "\n"
puts monster.print_scoreboard
:)
1 Answer
Brandon Keene
7,217 PointsFluffy is one cowardly monster:
-------------------------
Fluffy scoreboard
-------------------------
- Screams: 4
-------------------------
- Scares: 15
-------------------------
- Runs: 12
-------------------------
- Hides: 99
Rachelle Wood
15,362 PointsRachelle Wood
15,362 PointsHe is definitely the world's most terrifying monster.