Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial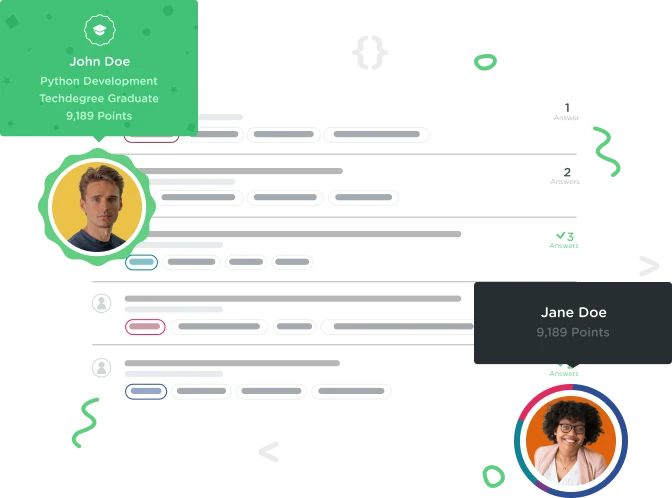

Raji Venkataraman
2,671 PointsI took "making an adventure story book" course of yours, i followed most of the steps though i had a different idea.
My idea was to tell a story and add a picture for each happening. The layout will have two buttons, Back and Next. It does not get input from the user. The code as such does not throw any error, but does not work the way i want it to. It shows me the first page. And when i click on the button, the code exits and does not go to the second as expected. I wrote a code based on what Ben taught me and i am attaching the code here.
This is the main class: package com.example.bharadwaj.storybook;
import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import android.widget.ImageView;
import android.content.Intent;
public class First extends AppCompatActivity { ImageView image; Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.first);
button = (Button)findViewById(R.id.first);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startStory();
}
});
}
private void startStory(){ Intent intent = new Intent(First.this, StoryActivity.class); startActivity(intent); }
@Override
protected void onResume() {
super.onResume();
}
}
Here is the StoryActivity class: package com.example.bharadwaj.storybook;
import android.content.Intent; import android.graphics.drawable.Drawable; import android.net.Uri; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import android.widget.ImageView; import android.widget.TextView;
import com.example.bharadwaj.storybook.model.Page; import com.example.bharadwaj.storybook.model.Story; import com.google.android.gms.common.api.GoogleApiClient;
public class StoryActivity extends AppCompatActivity {
public static final String TAG = StoryActivity.class.getSimpleName();
private Story mStory = new Story();
private ImageView mImageView;
private TextView mTextView;
private Button next;
private Button back;
private Page mCurrentPage;
/**
* ATTENTION: This was auto-generated to implement the App Indexing API.
* See https://g.co/AppIndexing/AndroidStudio for more information.
*/
private GoogleApiClient mClient;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.storyactivity);
mImageView = (ImageView) findViewById(R.id.storyimageview);
mTextView = (TextView) findViewById(R.id.StorytextView);
next = (Button) findViewById(R.id.Next);
back = (Button) findViewById(R.id.Back);
loadPage(0);
}
private void loadPage(int choice) {
mCurrentPage = mStory.getPage(choice);
Drawable drawable = getResources().getDrawable(mCurrentPage.getImageId());
mImageView.setImageDrawable(drawable);
mTextView.setText(mCurrentPage.getText());
if (mCurrentPage.isFinal()) {
next.setVisibility(View.INVISIBLE);
} else {
next.setText(mCurrentPage.getChoice1().getText());
back.setText(mCurrentPage.getChoice2().getText());
next.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getChoice1().getNextPage();
loadPage(nextPage);
}
});
back.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
}
This is Page activity(Model): package com.example.bharadwaj.storybook.model;
import android.graphics.pdf.PdfDocument;
/**
-
Created by Bharadwaj on 21/12/15. */ public class Page { private int ImageId; private String text; private Choice mChoice1; private Choice mChoice2; private boolean mIsFinal = false; public Page(int imageId, String mtext, Choice choice, Choice next){ ImageId = imageId; text = mtext; mChoice1 = mChoice1 ; mChoice2 = mChoice2; } public boolean isFinal(){ return mIsFinal; }
public void setIsFinal(boolean isFinal) { mIsFinal = isFinal; }
public int getImageId(){ return ImageId; } public void setImageId(int david){ this.ImageId = ImageId; }
public String getText() { return text; }
public void setText(String text) { this.text = text; }
public Choice getChoice1() { return mChoice1; }
public void setChoice1(Choice choice1) { mChoice1 = choice1; }
public Choice getChoice2() { return mChoice2; }
public void setChoice2(Choice choice2) { mChoice2 = choice2; } } Here is the Choice class: package com.example.bharadwaj.storybook.model;
/**
-
Created by Bharadwaj on 22/12/15. */ public class Choice { private String mText; private int mNextPage;
public Choice(String text, int NextPage) { mText = text; mNextPage = NextPage; }
public String getText() { return mText; }
public void setText(String text) { mText = text; }
public int getNextPage() { return mNextPage; }
public void setNextPage(int nextPage) { mNextPage = nextPage; } }
And the Story Class: package com.example.bharadwaj.storybook.model;
import com.example.bharadwaj.storybook.R;
/**
-
Created by Bharadwaj on 21/12/15. */ public class Story { private Page[] mPage;
public Story() { mPage = new Page[6]; mPage[0] = new Page( R.drawable.firstpage, "Once upon a time there where two countries that where arch enenmies, Israel and Philistine", new Choice("Go back",0), new Choice("Next", 2)
); mPage[1] = new Page( R.drawable.david, "David was a small but a wise Isralite man, who decided to oppose Goliath who was a giant in appearance", new Choice("Go back",1), new Choice("Next", 2) ); mPage[2] = new Page( R.drawable.golliath, "Golliath was a huge, giant who stood about 9 feet from the ground", new Choice("Go back",1), new Choice("Next", 3) ); mPage[3] = new Page( R.drawable.charging, "Golliath saw David and started moving towards him slowly but steadily. He was shocked to see the sticks David had in his hand ", new Choice("Go back",1), new Choice("Next", 4) ); mPage[4] = new Page( R.drawable.savuadi, "David, was a great at playing slingshot. Had a great eyesight and never missed a goal. Now Golliath's head was his goal and did not miss it.", new Choice("Go back",1), new Choice("Next", 5) ); mPage[5] = new Page( R.drawable.lastpage, "Thus Israel won and people of the land rejoiced.", new Choice("Go back",1), new Choice("Next", 6) ); mPage[6] = new Page( R.drawable.moral, "Thus the moral of the story is that a clear aim is what it takes to win and not huge appearances", new Choice("Go back",1), new Choice("Next", 1) );
} public Page getPage(int pageNumber){ return mPage[pageNumber]; } }
Here is Main Activity.xml <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context="com.example.bharadwaj.storybook.First" tools:showIn="@layout/activity_first" android:contextClickable="false">
<ImageView
android:layout_width="match_parent"
android:layout_height="300dp"
android:id="@+id/firstpage"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_above="@+id/first"
android:src="@drawable/titlepage"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="STORY BEGINS HERE!"
android:id="@+id/first"
android:singleLine="true"
android:textColor="#3086ef"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"
android:layout_marginBottom="71dp"/>
</RelativeLayout> And the StoryActivity.xml <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context=".StoryActivity" tools:showIn="@layout/activity_second_page" android:background="#ffffff">
<ImageView
android:layout_width="match_parent"
android:layout_height="200dp"
android:id="@+id/storyimageview"
android:src="@drawable/firstpage"
android:adjustViewBounds="true"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"/>
<TextView
android:layout_width="400dp"
android:layout_height="100dp"
android:text="Golliath saw David and started moving towards him slowly but steadily. He was shocked to see the sticks David had in his hand "
android:id="@+id/StorytextView"
android:paddingLeft="15dp"
android:paddingRight="15dp"
android:paddingTop="15dp"
android:lineSpacingMultiplier="1.2"
android:layout_below="@+id/storyimageview"
android:layout_alignLeft="@+id/storyimageview"
android:layout_alignStart="@+id/storyimageview"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Next"
android:id="@+id/Next"
android:layout_above="@+id/Back"
android:layout_alignLeft="@+id/StorytextView"
android:layout_alignStart="@+id/StorytextView"
android:textColor="#3a8aec"
android:background="#ffffff"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Back"
android:id="@+id/Back"
android:layout_alignParentBottom="true"
android:layout_alignLeft="@+id/Next"
android:layout_alignStart="@+id/Next"
android:layout_marginBottom="65dp"
android:textColor="#3a8aec"
android:background="#ffffff"/>
</RelativeLayout>