Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial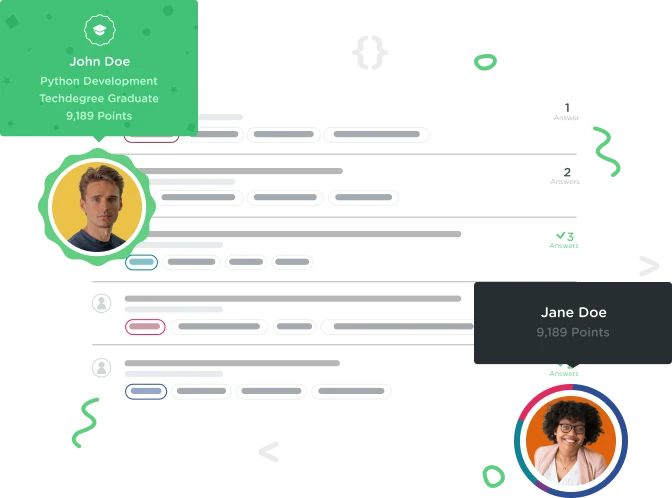

Kevin Smith
766 PointsI tried my own version of this code and I'm not sure what's wrong.
def trythis():
name = str(input("What is your name?"))
num1 = int(input("First number"))
num2 = int(input("Second number"))
answer = (num1 + num2)
return(answer)
print("Hello {}, your answer is {}".format(name, answer))
This is what I wrote into my Eclipse IDE, and the two errors that I am getting are: Unused variable: name - line 2 Undefined variable: name, answer line 7.
I'm confused as to why none of my prompts are showing up in the console. I'm also confused why "name is an unused variable". Kenneth used "age = str(input("What is your age")) and it worked.
I'm attempting to write some of my own code to see how much I have learned. I would like to know, conceptually, why I am making these errors. I feel like I'm still not getting something fundamental.
4 Answers
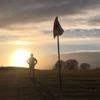
Stuart Wright
41,120 PointsThis relates to scope - i.e. the variables you've declared inside your function can only be accessed inside the function.
Here are a couple of possible solutions:
def trythis():
name = str(input("What is your name?"))
num1 = int(input("First number"))
num2 = int(input("Second number"))
answer = (num1 + num2)
print("Hello {}, your answer is {}".format(name, answer))
trythis()
This first option just moves your print statement inside the function so that it can access the name and answer variables.
def trythis():
name = str(input("What is your name?"))
num1 = int(input("First number"))
num2 = int(input("Second number"))
answer = (num1 + num2)
return name, answer
name, answer = trythis()
print("Hello {}, your answer is {}".format(name, answer))
If you want to access name and answer outside the function, you have to return them, then assign the result to global variables (that's what I've done on the line before the print statement). You've probably not come across returning more than one variable from a function yet, so don't worry if you're not completely comfortable with this. The first option is probably the best one to go with for now.
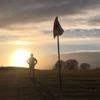
Stuart Wright
41,120 Pointstrythis() is the function call. It is you telling Python to run the code inside the function. If you run the script containing only a function definition and no call to run it, nothing will happen.
Return is used when you actually want to get some information out of the function (like I did in the second example above). But in the first example there's no need to since the print statement happens inside the function.
If you wanted to include name, num1 and num2 in the parameters of the function, it would look like this:
def trythis(name, num1, num2):
answer = (num1 + num2)
print("Hello {}, your answer is {}".format(name, answer))
name = str(input("What is your name?"))
num1 = int(input("First number"))
num2 = int(input("Second number"))
trythis(name, num1, num2)
Notice that your function now only contains two lines of code, but you pass values for name, num1 and num2 that you defined outside the function.

Kevin Smith
766 PointsHello, thanks for your reply! I am very relieved to see how close I was to blindly writing a functional piece of code. I have a few questions, however:
Why do I have to add "trythis()" at the bottom of the code (from python's perspective)? Why is it incorrect to include "return" and then "print". Is it just because it's redundant? Would it be wrong to include "name", "num1", and "num2" in the parameters of the function? If so, what are the correct parameters to include in a function?
Thank you again for your answer!!!

Kevin Smith
766 PointsThat actually makes perfect sense, thank you so much. Just one last question:
When would you want to leave the function without parameters, and when would you want to include them? What's the advantage to including a parameter?