Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial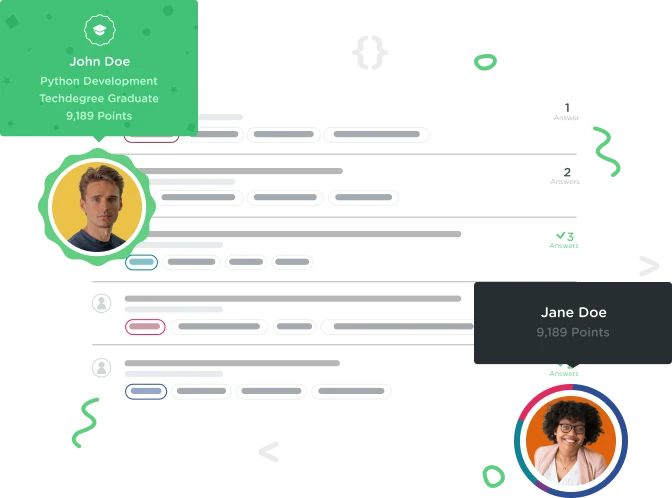

Lydia O' Brien
4,014 PointsI tried putting it all on one line
Can somebody show me how you got past this challenge? I am stuck on this one problem. It is the last challenge out of the three.
from django.shortcuts import render, get_object_or_404
from .models import Article, Writer
def article_list(request):
articles = Article.objects.all()
return render(request, 'articles/article_list.html', {'articles': articles})
def writer_detail(request, pk):
writer = Writer.objects.get(pk=pk)
return render(request, 'articles/writer_detail.html', {'writer': writer})
def article_detail(request, pk):
article = Article.objects.get(pk=pk)
return render(request, 'articles/article_detail.html', {'article': article})
def article_detail(request, pk):
try:
article = Article.objects.get(pk=pk)
except:
article = get_object_or_404(article, pk=pk)
return render(request, 'articles/article_detail.html', {'article': article})
from django.conf.urls import url
from . import views
urlpatterns = [
url(r'article/(?P<pk>\d+)/$', views.article_detail),
url(r'writer/(?P<pk>\d+)/$', views.writer_detail),
url(r'', views.article_list),
]
1 Answer

Ryan S
27,276 PointsHi Ellie,
You don't need to use try and except blocks to handle bad pk's if you are using the get_object_or_404()
function. It will handle that for you and return a 404 if the object doesn't exist.
When using get_object_or_404()
you will need to pass in the model you are querying. In this case it is "Article" (with a capital "A"). This is similar to using the Article.objects.get()
method where you are grabbing an instance of the model, except the model is passed in as an argument to the function.
The view can be reduced to the following:
def article_detail(request, pk):
article = get_object_or_404(Article, pk=pk)
return render(request, 'articles/article_detail.html', {'article': article})
Hope this helps.
Lydia O' Brien
4,014 PointsLydia O' Brien
4,014 PointsI tried that as well and even tried to change it, but the challenge is still not passing :( It says that Task 1 is no longer passing.
Ryan S
27,276 PointsRyan S
27,276 PointsDid you make sure to delete the other instance of your article_detail view? Initially I was just looking at your last view you defined in your code and didn't notice that you actually have 2 article_details.
In task 3 the challenge is basically asking you to rewrite article_detail, not add a new one. If you have two views with the same name it will definitely cause an error since django won't know which one you want to call.
Lydia O' Brien
4,014 PointsLydia O' Brien
4,014 PointsI did delete the other article_detail, I think it may be a glitch.
Ryan S
27,276 PointsRyan S
27,276 PointsIt is not likely that it is a glitch. The code is passing for me.
Could you post all your code from Task 3? There might be a little syntax error or something.
Lydia O' Brien
4,014 PointsLydia O' Brien
4,014 PointsI have my code on a different question, the title is I Tried Putting It All In One Line Part 2