Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial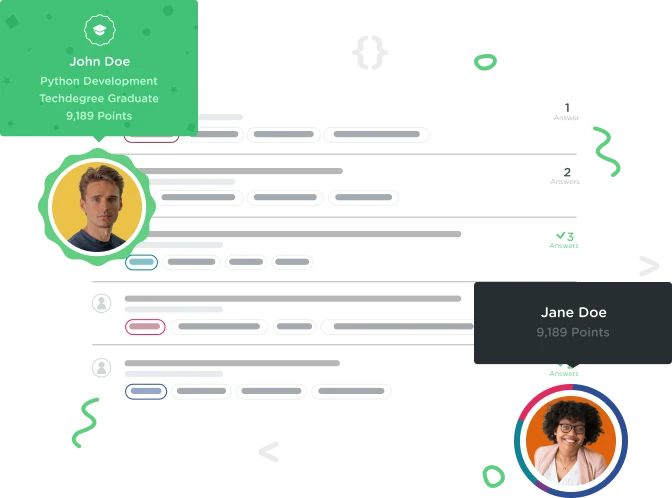

Sandy Woods
1,082 PointsI tried to compile my code and run it on the repl but had symbol not found errors
public class Game{
private String mAnswer;
public Game(String answer){
mAnswer = answer;
mHits ="";
mMisses = "";
}
//Public method will return true for a hit and false for miss/allow us to apply a guess
public boolean applyGuess (char letter){
boolean isHit = mAnswer.indexOf(letter) >=0;
//if true, we'll increment our hit variable.....else: if false, e
if (isHit){
mHits += letter;
}
else {
mMisses += letter;
}
return isHit;
}
}
[MOD: added ```java formatting -cf]
5 Answers
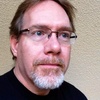
Chris Freeman
Treehouse Moderator 68,423 PointsYour code is very close. The issue is the variables assigned in the constructor have not been declared:
public class Game{
private String mAnswer;
private String mHits = ""; //<-- Added declaration
private String mMisses = ""; //<-- Added declaration
public Game(String answer){
mAnswer = answer;
mHits ="";
mMisses = "";
}
//Public method will return true for a hit and false for miss/allow us to apply a guess
public boolean applyGuess (char letter){
boolean isHit = mAnswer.indexOf(letter) >=0;
//if true, we'll increment our hit variable.....else: if false, e
if (isHit){
mHits += letter;
}
else {
mMisses += letter;
}
return isHit;
}
}

Sandy Woods
1,082 PointsThanks for responding Chris. I really appreciate it. Ok, so I made the corrections you suggested and even replaced my Game.java code w/ the code you corrected and received a cannot find symbol: class Game error. Do you have any idea what's going on there?
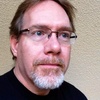
Chris Freeman
Treehouse Moderator 68,423 PointsThis is how it runs for me in the java-repl:
$ java-repl
Welcome to JavaREPL version 292 (Java HotSpot(TM) 64-Bit Server VM, Java 1.8.0_66)
Type expression to evaluate, :help for more options or press tab to auto-complete.
java> :load Game.java
Loaded source file from Game.java
java> Game game1 = new Game("apple")
Game game1 = Game@7b91e7d9
java> game1.applyGuess('a')
java.lang.Boolean res1 = true
java> game1.applyGuess('b')
java.lang.Boolean res2 = false
java>

Sandy Woods
1,082 PointsThat error was in the repl btw

Sandy Woods
1,082 PointsThanks for you help again Chris but I'm still unable to get up and running in the Repl in the way Craig Dennis did or you. I typed the same things in that you did for your code in my code. I'll probably re-visit the issue at a later date.
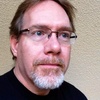
Chris Freeman
Treehouse Moderator 68,423 PointsCan you please repost your code and also the output from your repl session? Are you running in a workspace or locally on your own machine?

Sandy Woods
1,082 Pointspublic class Game{
private String mAnswer;
private String mHits = ""; //<-- Added declaration
private String mMisses = ""; //<-- Added declaration
public Game(String answer){
mAnswer = answer;
mHits ="";
mMisses = "";l
}
//Public method will return true for a hit and false for miss/allow us to apply a guess
public boolean applyGuess (char letter){
boolean isHit = mAnswer.indexOf(letter) >=0;
//if true, we'll increment our hit variable.....else: if false, e
if (isHit){
mHits += letter;
}
else {
mMisses += letter;
}
return isHit;
}
}
public class Prompter{
}
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game ("treehouse");
}
}
Treehouse Workspaces
Loaded source file from Game.java
java>Game game1 = new Game ("treehouse")
java.util.NoSuchElementException
[MOD: added ```java formating -cf]
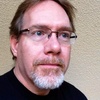
Chris Freeman
Treehouse Moderator 68,423 PointsIs there an extra trailing character on line 9? looks like an lower case L. Or is that a cut-and-paste error?
Sandy Woods
1,082 PointsSandy Woods
1,082 PointsHere's my Hangman code