Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial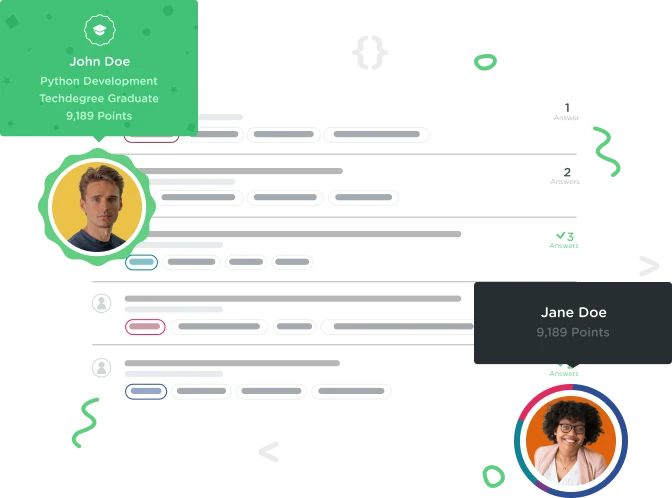
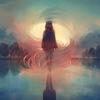
Aananya Vyas
20,157 Pointsi tried using codes online to get help and through documentation m unable to use this please help?
Next, let's add functionality to use the queue. First, create an instance method named enqueue that takes an element and adds it to the end of the Queue. Omit the external argument label here. For example, given the queue: [1,4,9], calling enqueue with 7, results in [1,4,9,7]
thats the question^ (My code below)
struct Queue<Element> {
var array: [Element] = []
var count : Int {
return array.count
}
var isEmpty: Bool {
if array.count == 0 {
return true
} else {
return false
}
}
class Node : Element {
// variables
var data: Element
var prev: Node?
init(data: Element){
self.data = data
}
}
// variables
var head: Node?
var tail: Node?
func enqueue(e: Element){
if head == nil && tail == nil {
head = Node(data: e)
head?.prev = nil
tail = head
} else {
var temp = Node(data: e)
tail?.prev = temp
tail = temp
}
}
func dequeue() -> Element{
var result = head?.data
if head === tail {
head = nil
tail = nil
} else {
head = head?.prev
}
if (result == nil){
return "empty"
}
return result!
}
}
1 Answer
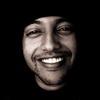
miikis
44,957 PointsHi Aananya,
You could have just asked for clarification on your earlier question, instead of creating a new one. Nonetheless, the reason you're having trouble with the Challenge is because you're over-complicating the tasks assigned.
You seem to be trying to implement a Linked-List but all the Challenge is asking you to do is to create a wrapper-struct around the array type so that the resulting structure behaves like a Queue (a First In, First Out structure). So:
- Your isEmpty property can just return array.isEmpty
- Your count property can just return array.count
- Your enqueue method can just array.append(element)
- Your dequeue method can just return array.removeFirst()
There's no need to create a Node class or mess around with head and tail properties. Let me know if that makes sense.
Aananya Vyas
20,157 PointsAananya Vyas
20,157 Pointsswift_lint.swift:23:18: error: cannot invoke 'append' with an argument list of type '((Element).Type)' return array.append(Element) ^ swift_lint.swift:23:18: note: expected an argument list of type '(Element)' return array.append(Element) ^
it shows this in the preview
miikis
44,957 Pointsmiikis
44,957 PointsYou forgot an argument label:
Aananya Vyas
20,157 PointsAananya Vyas
20,157 Pointsoops my bad thanks for the help though!