Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial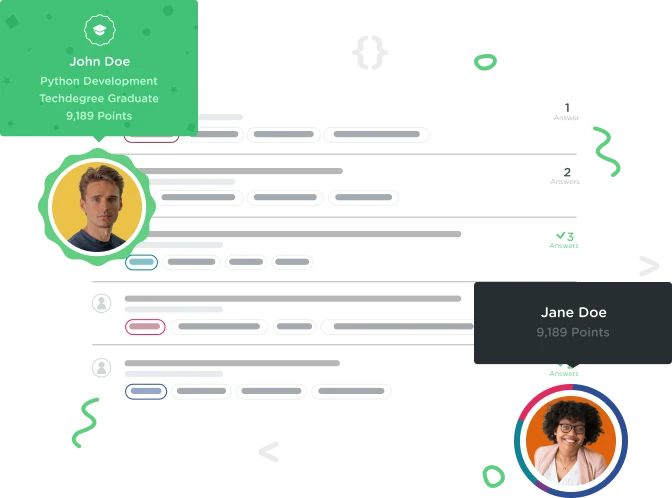

nicolaspeterson
8,569 PointsI tried using floats but couldn't figure out how to round the answer.
Imagine you have a list of prices that aren't round numbers (whole integers). How can I modify the code from the video to print out a rounded number?
2 Answers
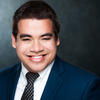
Jonathan Fernandes
Courses Plus Student 22,784 PointsTo piggy back off of Chris, use the python method round()
to round. It follows the conventional rules of rounding. So a number less than 5 at the tenth decimal place will round up and anything below will round down. Use it like this:
my_float_number = 3.49999999
my_other_float_number = 6.78475
new_number = round(my_float_number)
new_number_two = round(my_other_float_number)
print(new_number)
# => 3
print(new_number_two)
# => 7
So yeah, the first number would round down to 3 while the second number would round up to 7. Let me know if you have any more questions.
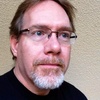
Chris Freeman
Treehouse Moderator 68,423 PointsHey Jonathan Fernandes, could you explain the origin of "a number less than 5 at the tenth decimal place"?
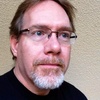
Chris Freeman
Treehouse Moderator 68,423 Pointsnicolaspeterson, the round is a bit more involved and has to do with the underlying binary representation. Floating point representation is inexact due to representing decimal numbers as binary. Python uses 53 binary bits to represent. Anything requiring a finer resolution will be lost.
For example, the closest binary representation of 2.675 is 2.67499999999999982236431605997495353221893310546875 which, as you can see will round down, not up, due to the "....49999999" part:
>>> round(2.675, 2)
2.67
Also, the decimal to binary conversion errors are not uniform. The error in 0.1 is not â…“ of the error in 0.3:
>>> 0.1 + 0.1 + 0.1 - 0.3
5.551115123125783e-17
>>> print('{:.50f}'.format(0.1 + 0.1 + 0.1 - 0.3))
0.00000000000000005551115123125782702118158340454102
Luckily there are libraries to handle when absolute precision is required. The fractions.Fraction object will keep track of rational number math without loss of precision. The decimal.Decimal object allows specifying the level of precision.
Using Decimal is crucial when working with dollar amount as fractional penny errors can add up significantly.
Good luck!!
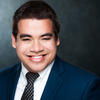
Jonathan Fernandes
Courses Plus Student 22,784 PointsHey Chris Freeman, sure, I can explain the statement "a number less than 5 at the tenth decimal place."
In my example, the number 3.4999999
would be rounded down because the number at the tenth decimal place is 4. That number is less than 5 so it will get rounded down. But if the number was 3.59999
, then the number at the tenth decimal place is now a 5, so it will be rounded up.
Long story short, I was just describing how the default functionality of the round()
method works without any other paramaters other than the number in question they wanted rounded.
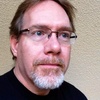
Chris Freeman
Treehouse Moderator 68,423 PointsJonathan Fernandes sorry, I misread your text as rounding depended on the “tenth” digit (as in after the ninth digit) and not as the “tenths digit position” (as in the first number after the decimal point).
So both our responds stand as correct! Yay!

nicolaspeterson
8,569 PointsThese are all great answers, thanks. I am aware of the round() function, I just wasn't entering it correctly. Simple syntax error.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsHave you look at the built-in round() function?