Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial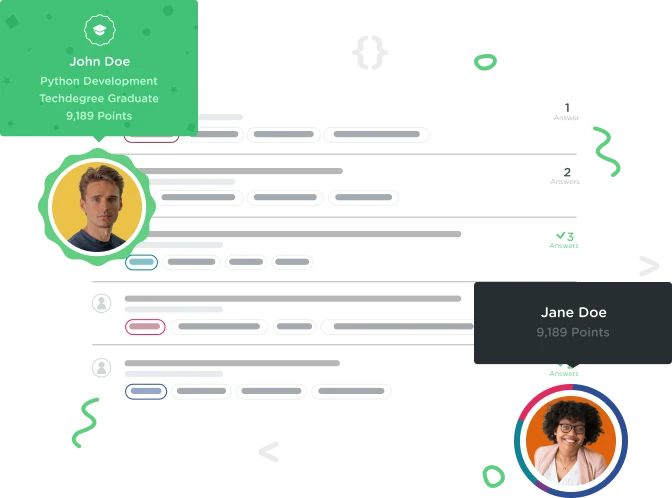

KORAXE IUHMAGEON
Courses Plus Student 8,486 PointsI typed parseInt() but it's giving me a parsing error
var correctGuess = false; var randomNumber = Math.floor(Math.random() * 6) +1; var guess prompt('I am thinking of a number between 1 and 6. What is it?'); if (parseInt(guess) === true) { alert('This is true'); } else { alert('This is false'); }
var correctGuess = false;
var randomNumber = Math.floor(Math.random() * 6) +1;
var guess prompt('I am thinking of a number between 1 and 6. What is it?');
if (parseInt(guess) === true) {
alert('This is true');
} else {
alert('This is false');
}
<!DOCTYPE HTML>
<html>
<head>
<meta charset="UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer

Thomas Nilsen
14,957 PointsA couple of things.
- You're missing an equals
var guess prompt('I am thinking of a number between 1 and 6. What is it?');
should be
var guess = prompt('I am thinking of a number between 1 and 6. What is it?');
This check is problematic
if (parseInt(guess) === true) {}
That will never be true because parseInt returns an int and it's being checked against a boolean using triple equals (===). When using triple equals, you saying that the value and type have to be equal.
You need to write
if (parseInt(guess) == randomNumber)
Notice the double equal (==), not triple.
Now, the number you entered through the prompt, is being checked against the randomly generated value.
here is a couple of examples to understand the difference between (==) and (===)
- == only checks the value
- === checks the type and value
console.log('1' == 1); //true
console.log('1' === 1); //false
console.log(1 == true); //true
console.log(1 === true); //false
console.log('' == false); //true
console.log('' === false); //false