Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial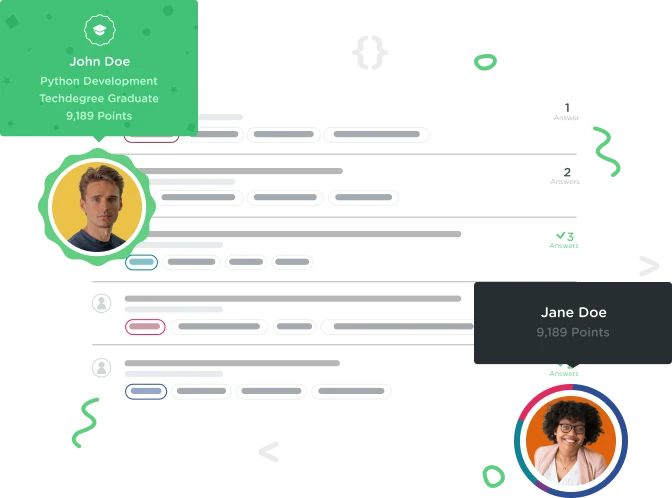
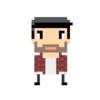
Scott Wells
8,715 PointsI understand callbacks, but I'm not understanding the purpose of passing an argument to a function.
I think I understand the usefulness of a callback - a function that is invoked when another function is invoked, either now or later?
However, for functions in general, I don't quite understand the purpose of an argument and how it relates to the function. The code from this lesson:
const div1 = document.getElementById('first');
const div2 = document.getElementById('second');
const div3 = document.getElementById('third');
function makeRed(element) {
element.style.backgroundColor = "red";
}
function makeBlue(element) {
element.style.backgroundColor = "blue";
}
function addStyleToElement(element, callback) {
callback(element);
}
addStyleToElement(div1, makeRed);
addStyleToElement(div2, makeBlue);
When the function makeRed is called, it changes the background color of the element to 'red'. But we never declared 'element' as a variable or defined what it was.
function makeRed(element) {
element.style.backgroundColor = "red";
}
Later in the code we have:
function addStyleToElement(element, callback) {
callback(element);
}
addStyleToElement(div1, makeRed);
I really have no idea what's going on here. The function 'addStyleToElement' inputs two arguments: 'element' and 'callback'. Then it reverses them or something weird when 'addStyleToElement' is invoked. Then the following line invokes 'addStyleToElement' again, but now it has different arguments? Does the order of the arguments matter? Does it affect what they do?
Is this essentially the same thing?
function addStyleToElement(x, y) {
y(x);
}
Thanks in advance. I've read all over the internet about this and I haven't found anybody that can example it for dummies.
3 Answers
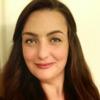
Jennifer Nordell
Treehouse TeacherHi there, Scott Wells ! There are already some brilliant answers left here, but I'd like to throw in my two cents if that's ok. The purpose of an argument is to give a value where there is none. When the function is defined, those parameters are essentially variables that have no value. Arguments are what we send into the function at the time we call it to give that parameter a value.
Imagine for a moment that you want to run a function on the last ten users to log in. Well, that list is going to change over time and depending on the traffic of your site, it might change very quickly. So we send in that list as it is right now and run that function.
I have, historically, used a PDF document that I wrote to illustrate the different parts of a function, their terminology, and why we need them. However, today I finally bit the bullet and posted it on Medium. It's a bit lengthy, but if you follow it to its conclusion, I feel like you will have a better understanding of arguments and perhaps functions in general. You can view the article here.
Hope this helps!
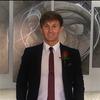
Liam Clarke
19,938 PointsHi Scott
The parameters/arguments you pass in a function are a way of using that information in a cleaner way, as well as allowing us to reuse the function multiple times.
These arguments can be named anything you like within a function.
function addStyleToElement( valueOfFirst, valueOfSecond ) {
valueOfSecond( valueOfFirst);
}
addStyleToElement( first, second );
So although the variable names are different, the values are passed to the argument.
This becomes very useful when using callbacks, Ajax, for example, you can pass in information to load different data based on the arguments you put in, thus allowing you to make one ajax function that can do multiple different requests.
Hope this helps! If not just let me know and il break down a more useful example of arguments and callbacks
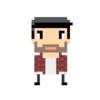
Scott Wells
8,715 PointsIn the original code I posted above, does the 'element' in...
function makeRed(element) {
element.style.backgroundColor = "red";
}
... share any relationship to the 'element' in ...
function addStyleToElement(element, callback) {
callback(element);
}
Or are they unrelated because they are in separate functions?
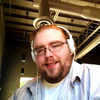
Matthew Griffith
21,003 PointsSo, on a basic level, 'element' is just a param that the function uses to get information to use within the function. What the words you use here does not matter. It could say:
function addStyleToElement(cats-and-dogs, donkey-kong) {};
What does matter is that you consistently use them throughout the function.
When you call ...
addStyleToElement(div1, makeRed);
...your function basically takes the info in the div1 variable and inserts it into the param 'element'. Then it takes the function makeRed and inserts it into the 'callback ' param. But, because its a function, in calls the makeRed function. The makeRed function takes the div1 info and process it.
In this case, the line with callback(element) is the equivalent to:
document.getElementById('first').style.backgroundColor = "red";
thus, changing the background of the element with the id 'first' to the red.
Callbacks allow you to recycle code.
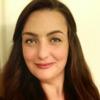
Jennifer Nordell
Treehouse TeacherHi there, Matthew Griffith1 I took the liberty of changing your "comment" to an answer. Thanks for helping out in the Community!
Scott Wells
8,715 PointsScott Wells
8,715 PointsThank you! Your article cleared up my confusion with the different parts of a function and what they do. Also, now I want a cheeseburger and onion rings. Cheers!