Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial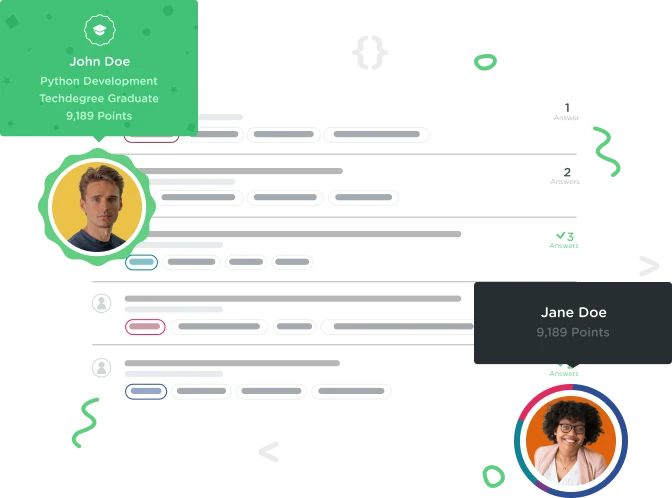
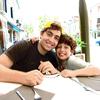
Daniel Ahmadizadeh
1,979 PointsI understand the video well, but I was wondering what the real world applications of using Hash's were. Thanks!
I understand the point of Arrays, but not so much Hash's.
3 Answers
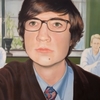
Steven Hicks
14,946 PointsAs quoted by http://ruby-doc.org/core-2.2.0/Hash.html A Hash is a dictionary-like collection of unique keys and their values. Also called associative arrays, they are similar to Arrays, but where an Array uses integers as its index, a Hash allows you to use any object type.
Here is an example of an array of hashes. Let's say you've created an instance variable @collections = Collections.all
and when you call @collections
you receive an array that contains hashes that looks something like this
{ state_code: "CA",
state: "California",
capital: "Sacramento",
timezone: "PST" },
{ state_code: "TX",
state: Texas,
capital: "Austin",
timezone: "CST" },
{ state_code: "NY",
state: "New York",
capital: "Albany",
timezone: "EST" }
]```.
Now, if you were to have a website that dynamically loaded this information based upon what page you were on, e.g. www.states.com/TX, the correct information could be loaded up. There are plenty more courses on the Ruby on Rails track that can show you more in depth how this is can be used.

Alexander Revell
5,782 PointsHi Darian, Hashes are useful for keeping track of items by name rather than by index – it's much easier to see and be specific about what you're trying to find when you can use the actual term, with keys and values that are easy to retrieve.
Particularly I've found when you start sending and receiving data through requests to servers, using forms, and most browser-related data transferral (although this is most often in JSON, but it's really similar)
It makes it so that you can access attributes of the hash object just like they are methods that return a value eg:
cat = {
name: "missy",
favourite_food: "biscuits"
}
# so then if you go
cat.name # => it should return "missy" to you
# which to me is a bit clearer than if it was an array and you had to say
cat[0] # => to have it return its name, "missy"
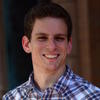
Jeff Lange
8,788 PointsOne of the most common uses of hashes you're going to end up seeing are objects you look up in the database (using the rails console).
For example, if you create a Rails web app, you are probably going to have a bunch of users you need to store in the database, right? Information will be stored about the users as hashes. You'll type something like "User.first" and it will give you a hash that looks something like this:
id => 1
name => "Joe Cool"
job_type => "Freelancer"
rate_per_hour => 85.00
onboarded_at => June 1, 2015
star_rating => 5
first_review => "Joe did a great job! We really enjoyed using him."
second_review => nil
etc, etc. The keys (e.g. name, job_type, etc) are the names of your columns in the database. The values (e.g. "Joe Cool", "Freelancer") are the specific values for the specific user, Joe Cool.
The columns (keys) would have been created by using Rails migrations as you developed the app. For example, "rails generate migration AddStarRatingToUsers star_rating:integer" followed by "rake db:migrate" would add the star_rating column as an integer to your User object in the database.
The values would be assigned as people use your app. For example, when Joe Cool signed up to your website, he filled out a form that asked for his name, job type, and hourly rate. When he submitted the form, those values were recorded in the database. Later, someone uses Joe and then when they use a form to review his work, they entered in a review and a star rating. When they submitted that form, those values were then recorded for Joe.
If a piece of information has never been entered yet, the value will be nil (i.e. the value for second_review in the example above).
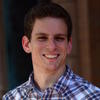
Jeff Lange
8,788 PointsIt's really easy to look up or call information on that object. For example, if in your app you need to dynamically generate a User's name, you can just type:
@user.name
By typing that you're saying, "For the current user, go into that User's hash and look up the :name key, then give me the value of the :name key." And if we were looking at Joe Cool's page, it would automatically generate, "Joe Cool", since that is the value of they key :name.
This is possible since Users are stored as hashes rather than Array's.
Daniel Ahmadizadeh
1,979 PointsDaniel Ahmadizadeh
1,979 PointsThis is great. Thanks so much Steven Hicks !