Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial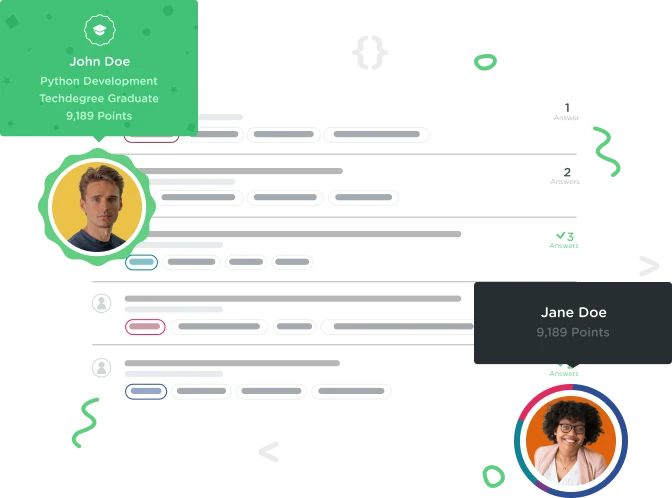

Alex Avila
8,570 PointsI used a function and if-else statement instead of a while loop.
Is it better to use a while loop instead of a function that acts like a loop with an if-else statement?
I changed the variables and strings to match the solution in the video, but this was my solution:
shopping_list = []
def add_items():
new_item = input("New item: ")
if new_item == "DONE":
print("\n".join(shopping_list))
else:
shopping_list.append(new_item)
add_items()
print("What should we pick up at the store?")
print("Enter 'DONE' to stop adding items")
add_items()

Alex Avila
8,570 PointsI call the function add_items() at the last line to start the function to add an item. I call it again after if/else to start the function again to add another item. This continues to happen until new_item == "DONE". If I didn't call the function again inside the function, after the if/else statement, I would only be able to add one item.
I used a recursive loop instead of a while loop because it's the first thing that came to my mind and I wanted to see if it was possible. Although, It would've probably been better to use a while loop for readability.

Keith Ostertag
16,619 PointsAlex- thanks for sharing! Reminded me to play around with something similar... and playing around is the way to learn!
3 Answers
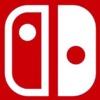
Cross Ender
9,567 PointsThat's an interesting idea, but I believe it is better to use the while loop. I like the use of recursion (functions calling themselves) in this example. The while loop is easier, in my opinion, to read.
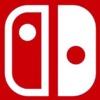
Cross Ender
9,567 PointsIf you like this idea, (I certainly do), then shopping_list should be a global variable. Do this by having your first line of code being "global shopping_list" to declare a global variable named shopping_list, then assign that variable an empty list.

Alex Avila
8,570 PointsThank you for your response. I read more about global variables after reading your response. I get the gist of it, but I still don't know what the purpose of using shopping_list as a global variable would be in this script. Would it be to avoid errors if I try to assign shopping_list a different value within the function, like 'shopping_list = 4', for example?
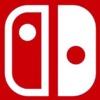
Cross Ender
9,567 PointsHere's the gist of scope: variables that are in a certain scope only exist in that scope. Global variables can be read and changed in any scope. Without the line "global shopping_list", shopping_list would only exist in the outermost part of the program, resulting in a possible traceback or logical errors. The reason is to avoid a Scope Error.
Sachin Kanchan
564 PointsSachin Kanchan
564 PointsWhy is the add_items() function called twice, once after if/else and again at the end? Regards