Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial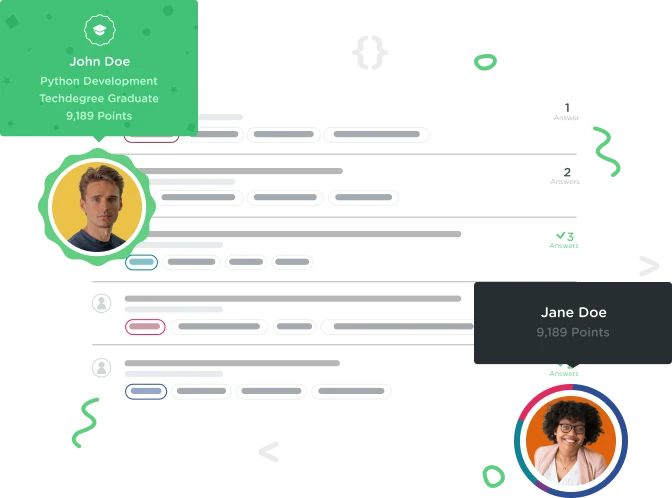

Myles Young
Full Stack JavaScript Techdegree Graduate 22,056 PointsI used functional components and props to pass around all values needed for this exercise. Worked out pretty good 👌🏽
Following the pattern of passing props from child to parent to manage state.
import React from 'react';
import PropTypes from 'prop-types';
export default function CrownLogo (props) {
const {highlight} = props;
return (
<svg viewBox="0 0 44 35" className={highlight}>
<path d="M26.7616 10.6207L21.8192 0L16.9973 10.5603C15.3699 14.1207 10.9096 15.2672 7.77534 12.9741L0 7.24138L6.56986 28.8448H37.0685L43.5781 7.72414L35.7425 13.0948C32.6685 15.2672 28.3288 14.0603 26.7616 10.6207Z" transform="translate(0 0.301727)" />
<rect width="30.4986" height="3.07759" transform="translate(6.56987 31.5603)" />
</svg>
);
}
CrownLogo.propTypes = {
highlight: PropTypes.string,
}

Myles Young
Full Stack JavaScript Techdegree Graduate 22,056 PointsTo get the highest score i just use the reduce method on the players array held in state to check if the current number is greater than the last largest number
let getHighScore = players.contestants.reduce((previousLargestNumber, currentLargestNumber) => {
return (currentLargestNumber.score > previousLargestNumber) ? currentLargestNumber.score : previousLargestNumber;
}, 0);
then just ship out that value as a prop
<Player
...
highScore={getHighScore}
...
/>
1 Answer

Myles Young
Full Stack JavaScript Techdegree Graduate 22,056 Pointsinside the player component i Import the CrownLogo and have a special function that determines what the "highlight" prop will be. it must be a string is defined in my PropTypes.
<CrownLogo highlight={highlight} />
Myles Young
Full Stack JavaScript Techdegree Graduate 22,056 PointsMyles Young
Full Stack JavaScript Techdegree Graduate 22,056 Pointsto determine if the "is-high-score" class is to be applied to the CrownLogo svg, I performed a check to see if my high score prop (passed in from parent component) is greater than the score of the rest of the players (values also passed from parent component). Note that the parent class sends back a score via props for each player on the board. each time the score is changed, a check is performed to see who has the highest score. The "highScore" prop references a callBack function that handles that logic.