Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial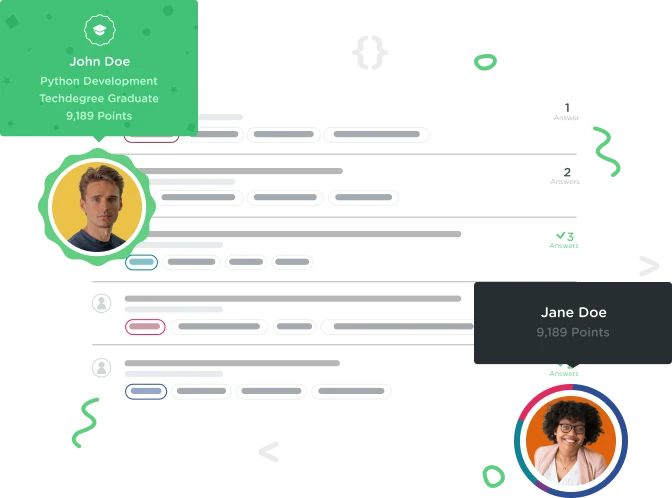
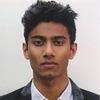
Samuel Kleos
Front End Web Development Techdegree Student 13,728 PointsI used the spread operator, and it came up with output on multiple lines?
I 'iterated' over this 2-dimensional array playlists
, looking to extract the second item (the artist) for every song in the array using a for loop.
My Code:
const playlist = [
['So What', 'Miles Davis', '9:04'],
['Respect', 'Aretha Franklin', '2:45'],
['What a Wonderful World', 'Louis Armstrong', '2:21'],
['At Last', 'Ella Fitzgerald', '4:18'],
['Three Little Birds', 'Bob Marley and the Wailers', '3:01'],
['The Way You Look Tonight', 'Frank Sinatra', '3:21']
];
const myArtists = (playlist) => {
for ( i=0; i<playlist.length; i++ ) {
let artists = [];
artists.push(playlist[i][1]);
}
console.log(...artists);
};
When I print to the console it comes out as a line-broken list, rather than a comma separated one:
> myArtists(playlist)
Miles Davis
Aretha Franklin
Louis Armstrong
Ella Fitzgerald
Bob Marley and the Wailers
Frank Sinatra
undefined
Also, how do I not get rid of the undefined
for the last item?
1 Answer
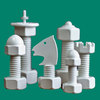
Steven Parker
231,275 PointsYou must have a globally defined "artists" created by code not shown here, because the one created inside the "for" loop is scope limited to within the loop and is not available to the console.log line after of it.
But even if it was, the multiple strings created by the spread operator would still not have comma separators, though they would appear on one line.
And the "undefined" you see is the natural result of calling a function in the console that has no return value (and myArtists doesn't return anything). The console always logs the result of anything you do manually.