Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial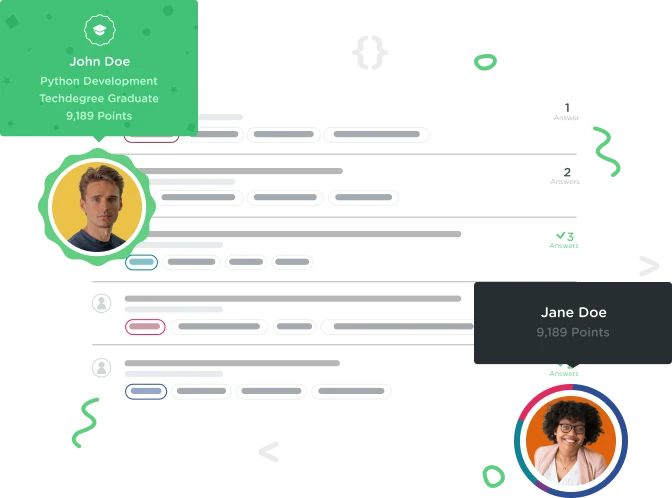
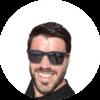
Daniel Muvdi
5,262 PointsI want to be clear about this interpretation.
I want to be clear about this. so because we are using the same random function to guess the number in our program we also could be using the same wrong numbers over and over in the attempts. i'm right? so the attempts could be even more than the total numbers available.
So in this case we could create a different function to guess faster the number by using only the numbers that are left after guessing.
6 Answers
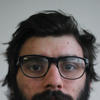
Zack Lee
Courses Plus Student 17,662 Pointsyes there is a chance that it could be guessing a single number multiple times, but with a range from 1 - 10000 it will be highly uncommon. also, to create a function that then has to compare each guess to all of its previous guesses would actually be slower, think if we ran the random number function to guess, and it guessed say 22, on its 3000th guess, it would have to compare 22 to an array of all 3000 guesses (thats a lot) then say it finds it has already guessed 22...well i guess we'll just ignore that guess? right a function like that would be paradoxical because the guess function has to fire before it can know if it already guessed that number. see what I'm saying? it actually faster to just brute force guess numbers regardless of if they've been guessed already.
to add to the point, random numbers aren't really random, they're pseudo-random, meaning the computer took a seed (say the current time) and ran it though a calculation to give the "random" number.
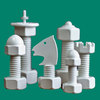
Steven Parker
229,744 PointsDaniel, you're absolutely right that the number of guesses can be more than the range of numbers due to duplicate guesses. But Zach also has a good point about how not to attempt to avoid them. So to efficiently guess without duplication requires a different strategy.
One way would be to create an array with a "pool" of potential guesses. Then each time you randomly choose an item from the pool and it is incorrect, you discard it and reduce the size of the pool. That way you can guarantee that the number of guesses will always be less than or equal to the total range, and every guess will require only one random choice.
var upper = 1000;
var randomNumber = getRandomNumber(upper);
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
var pool = [...Array(upper+1).keys()]; // create the guessing pool
pool.shift(); // but without 0
function nextGuess() {
var ix = getRandomNumber(pool.length); // get a random guess
return pool.splice(ix-1,1)[0]; // remove it from the pool
}
while (nextGuess() != randomNumber) {
attempts += 1;
}
document.write(`Computer took ${attempts}
attempts to guess the number: ${randomNumber}`);
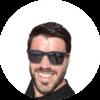
Daniel Muvdi
5,262 PointsHi guys, thanks for your answers but i think you can just add a line of code and make it a lot more faster. so instead of calling a function we just add += to guess number so each time the computer will try a bigger number until he finds it.
so is not need for an array or a big solution because we no need the old numbers we just need to make sure that we try them all.
please let me know what you think.
var upper = 1000;
var randomNumber = getRandomNumber(upper);
var guess = 0;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while (guess !== randomNumber) {
attempts += 1;
guess += 1;
}
document.write(" Computer took " + attempts +
" attempts to guess the number: " + randomNumber);
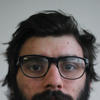
Zack Lee
Courses Plus Student 17,662 PointsFirst, you could remove the guess variable and just use "attempt" since they're always the same value. Second, this may be faster but defeats the purpose of guess random numbers. And this wont be faster in all cases. Say the number was 10000. The computer will make 10000 attempts until it "guesses" correctly. But if it was guessing random numbers this number could be significanlty less.
But yes, running through the numbers sequentially is a valid solution, i just think it defeats the purpose of what was trying to be demonstrated. i.e. the speed at which computers can perform a repetitive task with no clear end.
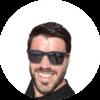
Daniel Muvdi
5,262 Pointsyes you can remove guess variable for attempts they have the same value, , and I agree that the propose of the exercise was the random numbers, my analysis was more for the fun of make it more efficient and practice my new knowledge. you are right about some times could be less faster than random but is guaranteed no bigger than the total numbers. so at the end you get a more efficient program (at least i think so lol).
so the question now is, is the program more efficient like this than with the random guessing? :)
Thanks!
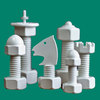
Steven Parker
229,744 PointsI added a code example to my answer. It always guesses in no more tries than the range, but totally unrelated to the number chosen. So unlike trying numbers in sequence, it might guess a high number in a small number of tries, but it could also take many guesses to get a small number.
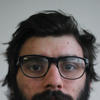
Zack Lee
Courses Plus Student 17,662 PointsYou could try implementing both ideas side by side and see which is more effecient.
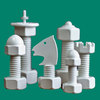
Steven Parker
229,744 PointsBoth methods average 500 guesses (half the range) to get the number, but the sequential method is much faster since it is essentially just a counter.
It can be made much faster still by maintaining just one counter:
do {
attempts += 1;
} while (attempts < randomNumber);