Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial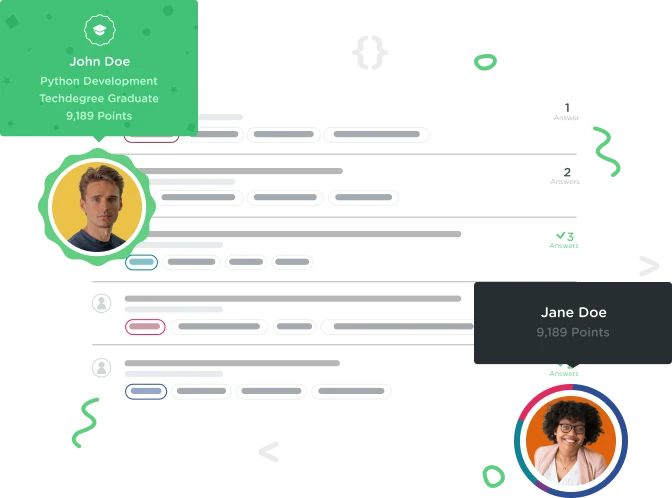

Benjamin Bradshaw
3,208 PointsI want to expand my knowledge
This code here works yet I didn't use slices like it recomended. I want to know how i would use slices so that i have a greater understanding of how and why it would work
def multiply(*args):
base = 1
for arg in args:
base = base * arg
return base
1 Answer

Jon Mirow
9,864 PointsHi there!
So first, I thought I should just say, you probably wouldn't - the way you a re doing it is readable, easy to maintain, doesn't require importing anything for one operation, etc. However, to answer the question, the way to do this with slice, would be by recursion. Basically you take the first element of the array, and then pass the rest of the array back into the function, which again takes the first element of the array, and passes the rest back in, until you have only one element in the array, then each function call returns the value it holds, multiplied by the value returned by function it called:
def multiply(*args):
def recursive_product(nums):
if len(nums) > 1:
return nums[0] * recursive_product(nums[1:])
else:
return nums[0]
return recursive_product(args)
Note that the recursive function is inside a wrapper function that appears to do nothing, This has the effect of reducing the args into one single argument so that the len() call works after the first recursion. You could do this by unpacking the list returned by slicing and passing into the next call with recursive_product(*nums[1:]), but no need to do that every single call!
Again, you wouldn't use this in real life, just because 1) You'd hit the recursion limit pretty fast if your had very long lists, and 2) the for loop is much, much faster, while using less memory. When I timed them for 1000 element arrays, recursion was slower by a factor of 3.75 on average. My testing code is here: https://pastebin.com/KeycpyM1
I'd be happy to explain anything if it's not clear, hope it helps :)