Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial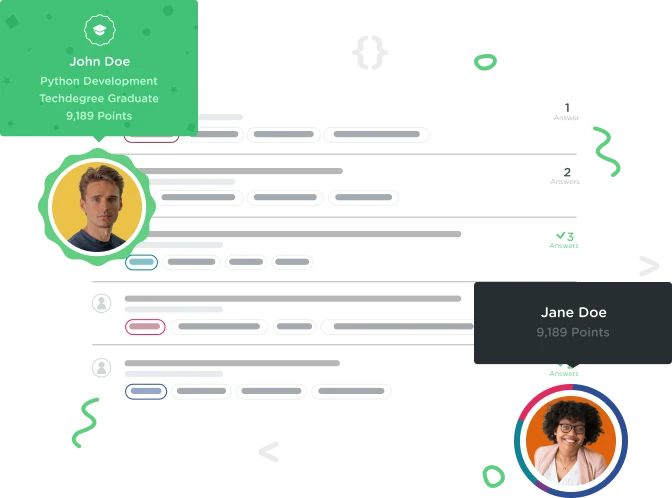

Burak Boysan
1,205 PointsI want to reach the input of a function.
I want to reach the input of a function but outside of the function.
For example;
func moneyBorrowed (#name: String) -> String? { let Borclular = ["Alex": "1000","David": "30"] for isim in Borclular { if isim.0 == name { return isim.1 } } return nil }
if let culprit = moneyBorrowed(name: "Alex"){ println(" (name) borrowed (culprit)£ ") }
this syntax doesn't work because name was not seen by the compiler out of the function. How can i make it work?
Please help
Thanks
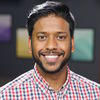
Pasan Premaratne
Treehouse TeacherFunction parameters can't be referenced outside of the scope of the function because they don't exist. What you can do is create a variable called name and store the name you are going to pass in, like so:
var name = "Alex"
if let culprit = moneyBorrowed(name: name) {}
Your code can also be simplified a bit, here's the final product I have:
func moneyBorrowed(#name: String) -> String? {
for (key,value) in ["Alex": "1000","David": "30"] {
if key == name {
return value
}
}
return nil
}
var name = "Alex"
if let culprit = moneyBorrowed(name: name) {
println("\(name) borrowed \(culprit)£")
}
Couple things, you don't need to store the dictionary in a constant, i.e. Borcular, to be able to iterate over it in a for loop. The loop can access the dictionary directly.
Second, you can use a tuple to store the dictionary key and value directly so that inside your for loop you can just reference the keys and values without having to use dot notation. This also makes it more readable since you know its a dictionary key versus isim.0
Third, in the function you've defined name as an external parameter. External names don't mean that you can use that variable outside of the function scope, it's mostly intended for readability so that when using the function you get a clean human readable name, whereas inside the body, you can use something shorter for brevity. A good example given in the Swift docs:
func join(string s1: String, toString s2: String, withJoiner joiner: String)
-> String {
return s1 + joiner + s2
}
In this example when using the function, we see the param names listed as string
, toString
and withJoiner
but the implementation of the function uses s1
, s2
and joiner
.
Finally, to use variables within a string like in your println statement, you need to use string interpolation. The variable/constant needs to be inside a set of empty parentheses with a preceding backslash, like so:
println("\(name) borrowed \(culprit)£")
Hope this helps!
Burak Boysan
1,205 PointsBurak Boysan
1,205 PointsI am sorry about the layout. I made it flat but it came out this way.