Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial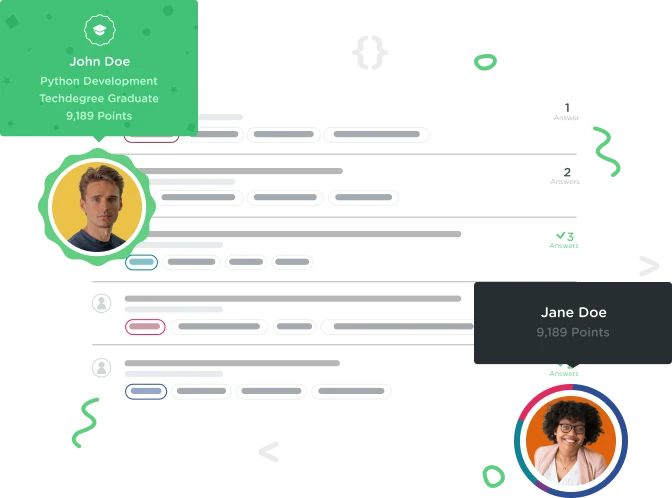

James Wood
9,804 PointsI want to track the mouse position and convert it into two different color stops for a gradient. can you help me?
I recently followed a tutorial not on treehouse where the code produces a rotating gradient background. I am working on a site for my portfolio that follows the story of someones near-death experience. There is a part of the story where the author explains colors being the entirety of his experience, constantly shifting and changing. I think this rotating background with its two color stops changing with user interaction would be a fitting effect for this part of my site.
//This is the code that produces the rotating background
var angle = 0;
var changeBackground = function () {
angle = angle + 1.3;
document.body.style.backgroundImage = "linear-gradient(" + angle + "deg, #ff4141, #2727e6)";
requestAnimationFrame(changeBackground);
}
changeBackground();
I am thinking of maybe tracking the x and y values separately and somehow converting those into colors or treat the x and y together and use a different equation to achieve two different colors. (x-y = one color + x+y = the second color.)
Thank you to all and any that have any ideas that could help me. I am fairly new to code and I am stumped on how to make this idea a reality.
1 Answer
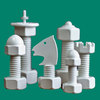
Steven Parker
230,274 PointsI was intrigued by the idea so I tried implementing it and came up with this:
// set background gradient colors and angle using mouse
document.onmousemove = e => {
var boxw = document.body.getBoundingClientRect().width;
var boxh = document.body.getBoundingClientRect().height;
var x = e.clientX - boxw / 2;
var y = e.clientY - boxh / 2;
var rad = Math.atan(y / x) + Math.PI * (x < 0 ? 1.5 : 0.5);
var deg = rad * 180 / Math.PI;
document.body.style.backgroundImage =
"linear-gradient(" + rad + "rad, hsl(" + deg + ",90%,30%), hsl(" + (deg + 120) + ",90%,30%))";
};
I also tried combining time-based color shifting with mouse-based angle changes. It wasn't as much fun to play with using the mouse, but it's more interesting by itself. You could possibly induce time-based changes only when the mouse has been still for a while.

James Wood
9,804 PointsWOW! Thank you very much! They don't lie in the videos when they say the community is helpful!
I am trying to break down this code so I can understand what is happening...
boxw = browser width boxh = browser height
x = the x position - the width and /2 y = the y position - the height and/2 Why is this equation used? Is this a go to for tracking position due to the value e.clientX/e.clientY returns?
rad (I assume short for radius) is an intimidating piece of code and I don't have a clue what is happening, I would be grateful if you could explain this to me.
deg (degrees?) is the value of the prior * 180 / math.PI (I assume is Pi 3.1415....) what does this equation achieve?
by looking at the linear gradient string you have used hsl() as the colors, what is this? (I am familiar with hex and rgb() and CMYK with print). (I also realise some of this I could google but I figure ill list everything I'm unfamiliar with anyway)
If I change the *180 I am guessing that would effect the rotation speed? and if I change the +120 that would be the differences in color.
I like the idea of shifting to a time-based effect after the mouse has been still for a while. I'd like to play around with this too.
Again Thank you very much!
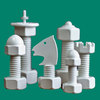
Steven Parker
230,274 PointsThis code simply computes the angle of a line from the center to the cursor, and uses that for the color choice and gradient direction. Here's a "glossary":
- x,y = coordinates with axis in center instead of upper left, for computing angle
- rad = angle in radians, the units returned by "atan"
- deg = angle converted to degrees, the units needed by "hsl"
- hsl = a different way to pick a color, Google it
- 180 is part of the rad->degree conversion, don't change that
- 120 is the anguar difference between the two color hues, play with that
James Wood
9,804 PointsJames Wood
9,804 PointsOr perhaps there is a better way to achieve a changing gradient that I have not anticipated?