Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial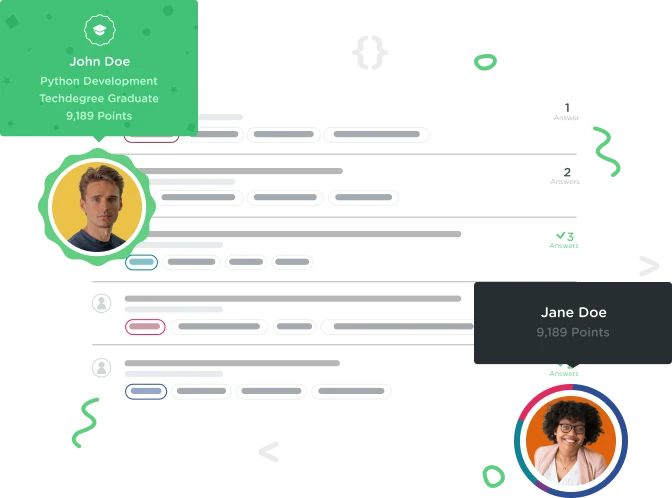
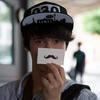
charlesmonaghan
742 PointsI was able to get this far, am I close?
Please don't give me the full answer, just tell me what I'm doing theoretically wrong :)
Any help is appreciated.
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
import random
def random_item(iterable):
return random.randint(0, len(iterable)) - 1
2 Answers
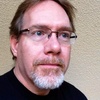
Chris Freeman
Treehouse Moderator 68,441 PointsYou are close. You've generated the required random number. Now use that number as the index to select an item from the iterable and return that item.
Also, you should probably put the "-1" inside the randint
argument with the len(iterable)
instead of subtracting 1 after generation the number. Otherwise, if the randint
was 0, you'd be left with a negative index (which would work but not for the reasons you want).
Post back if you need more help. Good luck!!
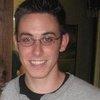
David Evans
10,490 PointsHi Charles,
So to not give the answer, I'll just draw your attention to the last line of the instructions.
Return the iterable member that's at your random number's index.
What you have done so far has gotten the index as random.randint(x,y) returns an integer. ( You will need to adjust your minus 1, its not quite in the correct place. )
You'll need to subtract one from the iterable, and not the random number you generate.
Once you fix the subtraction from the length of the iterable not the random number generated, keep in mind that you just generated an integer which will be your index and then you'll need to find the 'iterable member' or the single character that is associated with the number you generated.
charlesmonaghan
742 Pointscharlesmonaghan
742 PointsI did this. I'm kind of confused what I'm doing wrong haha. Do you know my issue?
David Evans
10,490 PointsDavid Evans
10,490 PointsHi Charles,
Rather than use the .index() try thinking of it this way.
Iterable is an array, so how would call a specific index of an array?
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsYou've moved the
-1
one paren too far. It should be outside theLen
function.Assign the result of the
randint
to a variable, such asindex
and use that variable as the index of the iterable in the formiterable[index]
.charlesmonaghan
742 Pointscharlesmonaghan
742 PointsGot it guys! Thank you so so much for the help, learnt heaps!
This was my final answer: