Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial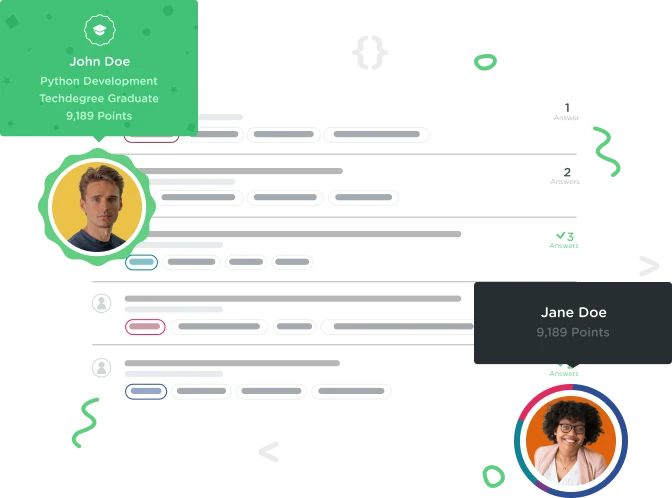

Mario Ocampo
192 PointsI was able to reach the same solution but my code is different.
name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
# TODO: Make sure the number is an integer
print("Hey!", name)
print("The number...", number)
# TODO: Print out the User's name and the number entered,
# making sure the two statements are on separate lines of output.
if number % 3 == 0:
print("is a Fizz number.")
elif number % 5 == 0:
print("is a Buzz number.")
elif number % 15 == 0:
print("is a FizzBuzz number.")
else:
# Just in case the number is neither div by 3 nor 5.
print("is neither a fizzy or a buzzy number.")
# TODO: Compare the number the user gave with the different
# FizzBuzz conditions.
# *********************
# If the number is divisible by 3, print "is a Fizz number."
# If the number is divisible by 5, print "is a Buzz number."
# If the number is divisible by both 3 and 5, print "is a FizzBuzz number."
# Otherwise, print "is neither a fizzy or a buzzy number."
# *********************
# TODO: Define variables for is_fizz and is_buzz that stores
# a Boolean value of the condition. Remember that the modulo operator, %,
# can be used to check if there is a remainder.
# Using the variables, check the condition of the value, and print the necessary
# string
So I did it this way and the result still came out the same as in the video. Is that strange how my code is different than Ken's yet both produced the same results?
3 Answers
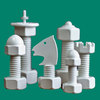
Steven Parker
231,275 PointsWhen posting code, use Markdown formatting to preserve the code's appearance (particularly indentation) and retain special symbols.
But this code doesn't seem like it would give the correct result for all numbers. Try it with 30, for example, which should be "a FizzBuzz number". To fix it you'll need to change the order the tests are performed in.

Mario Ocampo
192 PointsI tried it with 30 and it said 30 is a fizz number. I have run multiple times and it always gives me the correct answer.
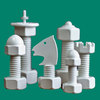
Steven Parker
231,275 Points30 should be "a FizzBuzz number". To fix it you'll need to change the order the tests are performed in and test for "FizzBuzz" first..

Mario Ocampo
192 Pointsname = input("Please enter your name: ")
number = int(input("Please enter a number: "))
# TODO: Make sure the number is an integer
print("Hey!", name)
print("The number...", number)
# TODO: Print out the User's name and the number entered,
# making sure the two statements are on separate lines of output.
if number % 15 == 0:
print("is a FizzBuzz number.")
elif number % 3 == 0:
print("is a Fizz number.")
elif number % 5 == 0:
print("is a Buzz number.")
else:
# Just in case the number is neither div by 3 or 5.
print("is neither a fizzy or a buzzy number.")
# TODO: Compare the number the user gave with the different
# FizzBuzz conditions.
# *********************
# If the number is divisible by 3, print "is a Fizz number."
# If the number is divisible by 5, print "is a Buzz number."
# If the number is divisible by both 3 and 5, print "is a FizzBuzz number."
# Otherwise, print "is neither a fizzy or a buzzy number."
# *********************
# TODO: Define variables for is_fizz and is_buzz that stores
# a Boolean value of the condition. Remember that the modulo operator, %,
# can be used to check if there is a remainder.
# Using the variables, check the condition of the value, and print the necessary
# string
I've got it! I fixed my code and now it displays 30 as a FizzBuzz number. Thanks for your help, it was the order in which the tests were done, that was the issue. I'm glad that I did not give up and kept trying and trying.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsNote that "a Fizz number" is not the correct answer for 30. Since 30 is divisible by both 3 and 5, it should be "a FizzBuzz number". To get the correct results, you'll need to test for "FizzBuzz" first.