Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial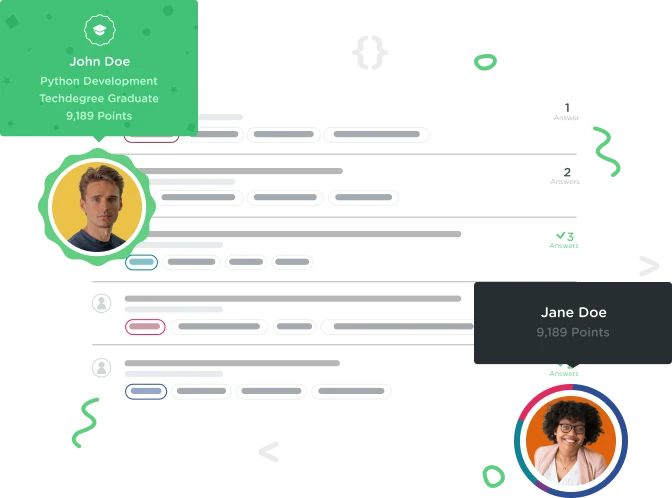
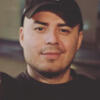
victor E
19,146 Pointsi was certain this was correct
not sure why my syntax is wrong.
var objects = [
var object_a = {object1: thing1, object2: things2},
var object_b = {object3: things3, object4: things4},
var object_c = {object3: thing5, object2: things5}
]
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Objects</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
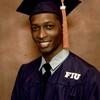
Dane Parchment
Treehouse Moderator 11,077 PointsIt should look like this:
var object_a = {object1: thing1, object2: things2};
var object_b = {object3: things3, object4: things4};
var object_c = {object3: thing5, object2: things5};
var objects = [
object_a,
object_b,
object_c
];
The problem is the following syntax:
var object_a = {object1: thing1, object2: things2};
Is what we call a variable declaration, you can't use a variable declaration as a (non-parameter) value. That is what is the syntax error you are running into. Instead, you must pass in the value of the declaration.
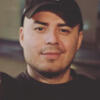
victor E
19,146 Pointsyeah they wanted me to just put the objects inside the array without defining them outside of it. the second example worked. thank you!
victor E
19,146 Pointsvictor E
19,146 Pointsthat makes a lot sense thank you!
victor E
19,146 Pointsvictor E
19,146 PointsI tried it and it didn't work :(
Dane Parchment
Treehouse Moderator 11,077 PointsDane Parchment
Treehouse Moderator 11,077 Pointsprobably because the variables
thing1, thing2, thing3, thing4, thing5
don't exist. I was just correcting your syntax, I assumed that you had already created and assigned values to those variables. Otherwise, it should look like this:or more concisely like so: