Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial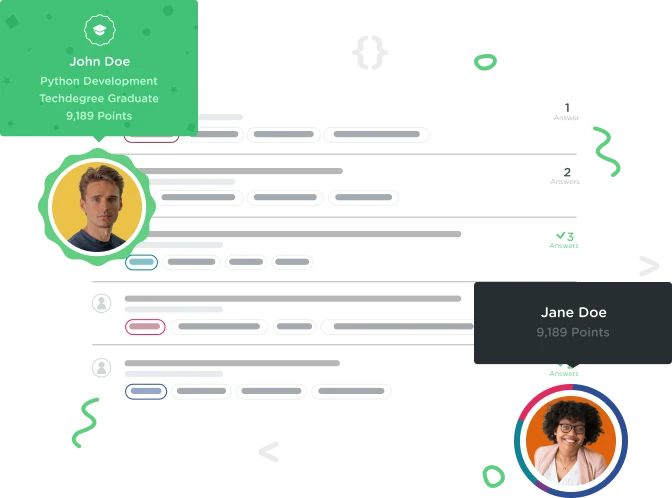

Aaron Coursolle
18,014 PointsI was following along until shorthand was introduced
This is a code that contains two conditional statements, but I'm only showing the second one below:
if (correctGuess === true) {
document.write('<p>You guessed the number!</p>');
} else {
document.write('<p>Sorry. The number was ' + randomNumber + '</p>');
}
This code makes sense to me, but in the shorthand:
if (correctGuess) {
document.write('<p>You guessed the number!</p>');
} else {
document.write('<p>Sorry. The number was ' + randomNumber + '</p>');
}
the code does not. How does it work if you aren't explicitly stating if correctGuess is true or false.
2 Answers

Geoff Parsons
11,679 PointsWhatever is inside of the parenthesis of the conditional is evaluated to true or false and that determines if that particular block of code is run. For instance you could ask if a variable has the value "tacos"
if ( food === "tacos" ) {
// do something
}
Which will evaluate food === "tacos"
to either true or false. If the variable you are checking already contains one of the literal values true
or false
then you do not need to use an equality operator (===
).
var myBoolean = true;
if ( myBoolean ) {
// code here will run
}
var otherBoolean = false;
if ( otherBoolean ) {
// code here will not run
}

Victoria Felice
14,760 PointsHi Aaron, I think that you got the main point. This is just a shorthand.
"if (correctGuess)" means "if (correctGuess === true)". In other words:
Longhand:
if (correctGuess === true)
Shorthand:
if (correctGuess)
I found a good article about JavaScript shorthands http://www.sitepoint.com/shorthand-javascript-techniques/ This particular case is mentioned there as well: paragraph 8. If Presence Shorthand. HTH.

Aaron Coursolle
18,014 PointsLooks like a very helpful article. Thank you.
Nick Hettinger
10,815 PointsNick Hettinger
10,815 PointsI don't understand how the program would know which code to run if the correctGuess variable can be either true or false (dependent on user input). For example, if the user guesses wrong, then the correctGuess value would stay false. In this case, what prevents the program from running the code that tells the user that their guess was correct?
Manuel Sanchez
8,971 PointsManuel Sanchez
8,971 PointsNick, that's why you set the variable correctGuess to false, so its value can only be set to true when the user guess is right. In other words, you're assuming that the user is wrong until its input proves the opposite. Making "hypotheses" beforehand is a common programming pattern. You'll see a lot of programming problems that can be solved by this "it's true until proven false" (and vice versa, but not that frequently) approach.