Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial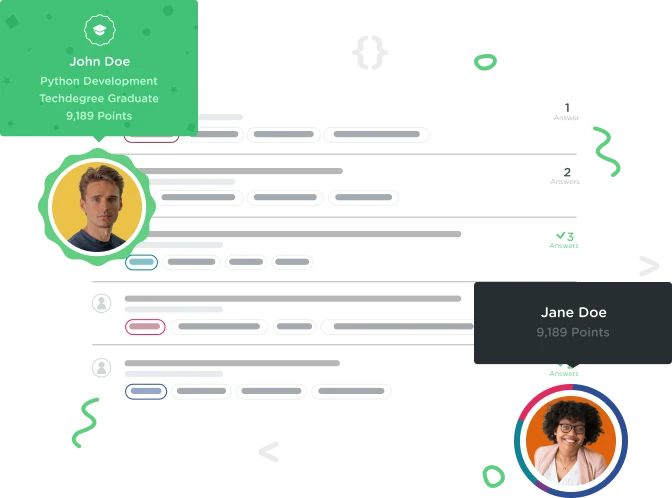

Russell Brookman
3,118 PointsI worked on this long enough. Does anyone know what I'm missing here?
Here is the problem it's giving me.
./TeacherAssistant.java:10: error: cannot find symbol if (! mName.isFieldName(fieldName)) { ^ symbol: method isFieldName(String) location: variable mName of type String 1 error
The question is to find and return an error. I have tried it with Charset.fieldName(fieldName); I have also tried it several other ways that I found on line. It just doesn't want to work.
public class TeacherAssistant {
private static String mName;
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
mName = fieldName;
if (! mName.isFieldName(fieldName)) {
throw new IllegalArgumentException("field name incorect");
return fieldName;
}
}
}
3 Answers

Micheal Ammirati
5,377 PointsA few things here:
- You don't need to create a new variable "String mName" you can simply use the fieldName that is passed into the method and perform the logic on that variable.
- You need to check for the 2 criteria given in comments. the first character of fieldName must be 'm' and the second character must be uppercase. This means you will need two logic checks (if statements)
- I'm not sure where you got the isFieldName() method from? you should be using charAt() to be checking the pertinent character in each if statement. Remember: in programming we start from 0.
- You have the return statement inside of your if statement. This means that if your if statement evaluates to false and gets passed over your method will never return! The IllegalArgumentException inside your if statement will throw an exception and break you out of the method so you won't need to add a return after it inside your if statement.
Hope that helps enough without giving too much away
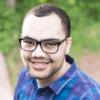
Philip Gales
15,193 PointsI think you over thought this challenge. It was just asking you to use an if statement (you did) to see if the first letter was an "m" and the second character was upper case. Instead of using negatives (!), I just used an else statement.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
//if both the first char is an m and the second char is uppercase then nothing, otherwise throws exception
if (fieldName.charAt(0) == 'm' && Character.isUpperCase(fieldName.charAt(1))){
//yay, the answer being tested (fieldName) fits the parameters and nothing happens
} else {
//fieldName did not meet our specifications and an error is thrown
throw new IllegalArgumentException("field name incorect");
}
//returns the value when it is correct. If it was wrong then the error would be thrown and the method would have
//been exited before reaching this statement.
return fieldName;
}
}

Russell Brookman
3,118 PointsBetween the 2 of you, and re-looking up how charAt works to get a better understanding, I think I got it. Thanks a lot for all of your help.
Philip Gales
15,193 PointsPhilip Gales
15,193 PointsWow! You gave so much more detail than I did. I generally give out the answers now because I first unsubscribed and quit Treehouse due to being unable to solve some of the challenges. I do not want anyone else to quit so I just give them a short description and answer and assume in the future they'll get an "ah ha" moment like I did.