Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial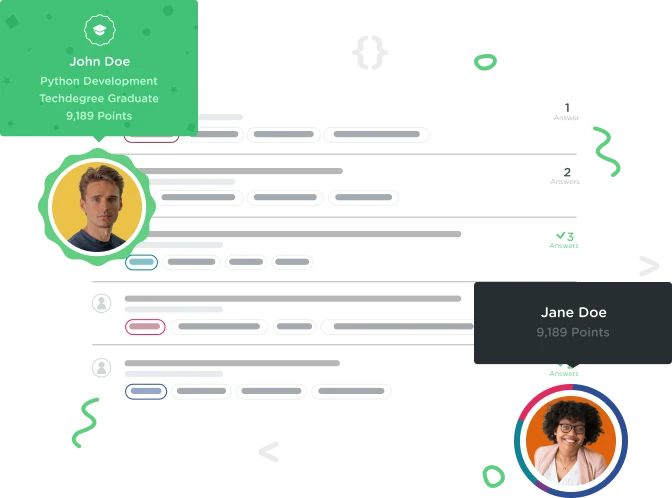

Michael Brown
17,651 PointsI would like feedback on my code, if possible please!
Hey everybody! I just finished working on this practice code for this workshop, and I'm quite proud that I got to work without looking up the answer. However, I have a question. I have seen some other people doing it a different way, and was basically just asking: What would be the BEST practice way to do this? Here is my code:
var name = prompt("What is your first name?");
var lastName = prompt("What is your last name?");
var fullName = name +" " + lastName;
console.log(fullName);
var lengthOfString = fullName.length;
console.log(fullName + " is " + lengthOfString + " characters long" );
3 Answers
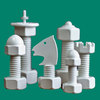
Steven Parker
241,783 PointsIf you have a question about a particular difference, post the other version for comparison.
Otherwise, one minor point is that you might want to avoid creating variables that are only used one time. So, for example, if "lengthOfString " is not used again after the message is logged, you could skip creating it and output the message like this:
console.log(fullName + " is " + fullNameInCaps.length + " characters long" );
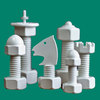
Steven Parker
241,783 PointsYour code seems functionally identical to the "official" solution except for the clever way you managed to call "toUpperCase" only one time.

Piotr Manczak
260 PointsWould it do the job?
const firstName = prompt('What is your first name?').toUpperCase();
const secondName = prompt('What is your second name?').toUpperCase();
alert(The length of your full name is: ${firstName.concat(' ', secondName).length} characters long.
);

Piotr Manczak
260 PointsI like it. As long as it works it's fine. I did something different using MDN.com. it is more condensed.
const firstName = prompt('What is your first name?').toUpperCase();
const secondName = prompt('What is your second name?').toUpperCase();
alert(The length of your full name is: ${firstName.concat(' ', secondName).length} characters long.
);
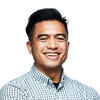
Neil Martin Orbase
4,137 PointsThat means so much, @Steven! Thank you so much for the quick feedback -- definitely keeps me motivated on my journey with coding and thinking like an engineer!
Neil Martin Orbase
4,137 PointsNeil Martin Orbase
4,137 Points@Michael Brown this was my solution: