Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial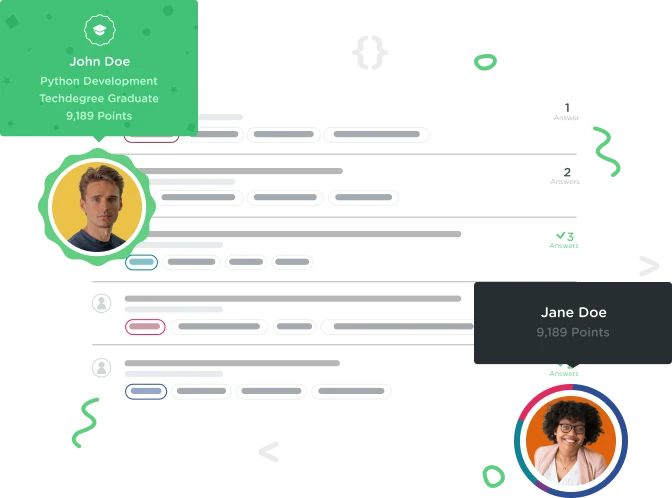

jl64
Full Stack JavaScript Techdegree Graduate 19,359 PointsI would like JavaScript Version of Everything in this course, and the Algorithm Courses.
The email provided doesn't work, and numerous students from various tracks, studying various languages, have been asking for these lessons in their language of choice for years now. I've seen staff only chime in to tell students to send the issue to the support staff. Several have claimed to have done so, and no effort has been made to remedy this pervasive issue.
1 Answer
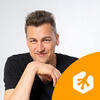
Erland Van Olmen
Full Stack JavaScript Techdegree Graduate 17,825 PointsI made a simple implementation of Merge Sort in JavaScript, hope it helps whoever wants something to play with. It is not optimized in any way though.
// function to fill an array 'list' with the amount 'numValues' of values
// between 0 and maxVal (excluding maxVal):
function fillUnsorted(list, numValues) {
const maxVal = 16;
for (let i = 0; i < numValues; i++) {
list.push(Math.trunc(Math.random() * maxVal));
}
return list;
}
function mergeSort(list) {
let listA = [];
let listB = [];
const len = list.length;
if(len > 1) {
const halfLen = Math.trunc(len / 2);
listA = mergeSort(list.slice(0, halfLen));
listB = mergeSort(list.slice(halfLen));
} else {
return list;
}
// merge both lists together:
let indexA = 0;
let indexB = 0;
let destList = [];
while (indexA < listA.length && indexB < listB.length) {
if (listA[indexA] < listB[indexB]) {
destList.push(listA[indexA]);
indexA++;
} else {
destList.push(listB[indexB]);
indexB++;
}
}
if (indexA < listA.length) {
destList = [...destList, ...listA.slice(indexA)];
} else if (indexB < listB.length) {
destList = [...destList, ...listB.slice(indexB)];
}
return destList;
}
let unsorted = [];
fillUnsorted(unsorted, 13);
console.log('Unsorted list: ', unsorted);
console.log('Sorted list: ', mergeSort(unsorted));
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsTo help your case, I will mark this post feedback. (A Mod can do this if the post links to the topic material. Thanks!)