Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial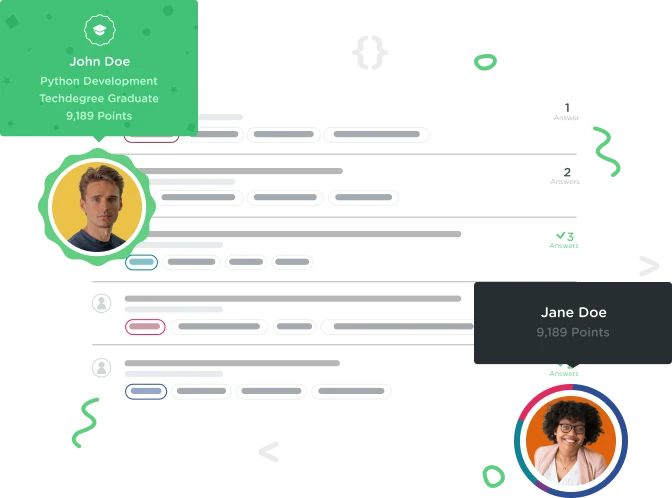
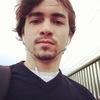
Leonardo Motta
11,284 PointsI would like to share my conditional challenge solution
my code generates 5 questions randomly.
here is the codepen link: https://codepen.io/leomotta/pen/bKyEaG
if someone tries the code tell me how many stars! :D
any feedback?
HTML5:
<!DOCTYPE html>
<html lang="en">
<head>
<script type="text/javascript" src="script.js"></script>
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.1.0/css/all.css" integrity="sha384-lKuwvrZot6UHsBSfcMvOkWwlCMgc0TaWr+30HWe3a4ltaBwTZhyTEggF5tJv8tbt" crossorigin="anonymous">
<link rel="stylesheet" href="css/style.css">
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<footer>
<p>All questions and answers were removed from <a href="http://www.funtrivia.com/en/Entertainment/Marvel-DC-6385.html">here.</a></p>
</footer>
</body>
</html>
CSS3:
p,
h1 {
text-align: center;
}
.fa-star {
color: #e5c100;
font-size: 40px;
}
#star p {
text-align: center;
}
JavaScript:
console.log('Start program');
/* GREETINGS, THIS IS THE MARVEL UNIVERSE QUIZ, ALL THE QUESTIONS AND ANSWERS WERE TAKEN AT: http://www.funtrivia.com/en/Entertainment/Marvel-DC-6385.html */
alert('Hi here goes five questions about Marvel/DC universe, good luck!');
// Generates a random number between 1 and 3 to pick a question.
var randomQuestion = Math.floor(Math.random() * 3) + 1;
console.log('random number question 1: ' + randomQuestion);
// The player starts with 0 score
var score = 0;
// Pick a question based on our RandomQuestion variable by using if and else statement.
/* ############################ FIRST QUESTION ############################ */
if (randomQuestion === 1) {
// option 1
var questionOne = prompt("One of Doctor Strange's greatest foes is the dread (not dreaded, just dread) Dormammu, who has gone through many costume alterations. What color was his original costume? (1/5)");
if (questionOne.toUpperCase() === 'GREEN') {
score++;
}
console.log('score: ' + score);
} else if (randomQuestion === 2) {
// option 2
var questionOne = prompt("Here are some facts about this famous superhero: Gamma radiation, very big, bad temper and his alias was Bruce Banner. He has had numerous television shows and movies featuring his character. Glen Talbot was a constant military nemesis of his. What is the name of this superhero? (1/5)");
if (questionOne.toUpperCase() === 'HULK') {
score++;
}
console.log('score: ' + score);
} else {
// option 3
var questionOne = prompt("A mysterious figure in purple appears in each of the first issues. She is often hidden and never speaks but is always watching. Who is she? (1/5)");
if (questionOne.toUpperCase() === 'PANDORA') {
score++;
}
console.log('score: ' + score);
}
/* ############################ SECOND QUESTION ############################ */
//New random number
randomQuestion = Math.floor(Math.random() * 3) + 1;
console.log('random number question 2: ' + randomQuestion);
if (randomQuestion === 1) {
// option 1
var questionTwo = prompt("What is the real name of the member of the Uncanny X-Men known as Nightcrawler? (2/5)");
if (questionTwo.toUpperCase() === 'KURT WAGNER') {
score++;
}
console.log('score: ' + score);
} else if (randomQuestion === 2) {
// option 2
var questionTwo = prompt("Batman's faithful butler, Alfred, has been with the Wayne family for quite a while, and as familiar as he is to comic books fans, do you know his last name? (2/5)");
if (questionTwo.toUpperCase() === 'PENNYWORTH') {
score++;
}
console.log('score: ' + score);
} else {
// option 3
var questionTwo = prompt("What is the Avenger known as Hawkeye's real name? (2/5)");
if (questionTwo.toUpperCase() === 'CLINT BARTON') {
score++;
}
console.log('score: ' + score);
}
/* ############################ THIRD QUESTION ############################ */
//New random number
randomQuestion = Math.floor(Math.random() * 3) + 1;
console.log('random number question 3: ' + randomQuestion);
if (randomQuestion === 1) {
// option 1
var questionThree = prompt('In "The Claws of The Cat", who was behind the mask of this feline-oriented superheroine? (3/5)');
if (questionThree.toUpperCase() === 'GREER NELSON') {
score++;
}
console.log('score: ' + score);
} else if (randomQuestion === 2) {
// option 2
var questionThree = prompt("1950 was the last year publisher Martin Goodman used the name \"Timely Comics\" to sell his line of comicbooks before changing its' name to Atlas Comics. Marvel Boy was the last character considered a superhero to have their own book until 1961. What planet did Robert Grayson receive the alien technology from to fight crime back on earth? (3/5)");
if (questionThree.toUpperCase() === 'URANOS') {
score++;
}
console.log('score: ' + score);
} else {
// option 3
var questionThree = prompt("This heroine has been a member of Justice League International. Her best friend is her teammate, Fire, and her boyfriend is the Green Lantern, Guy Gardner. She has the ability to shoot ice from her hands and was originally a princess. Who is this heroine? (3/5)");
if (questionThree.toUpperCase() === 'DR. OCTOPUS') {
score++;
}
console.log('score: ' + score);
}
/* ############################ FOURTH QUESTION ############################ */
//New random number
randomQuestion = Math.floor(Math.random() * 3) + 1;
console.log('random number question 4: ' + randomQuestion);
if (randomQuestion === 1) {
// option 1
var questionFour = prompt('In "The Claws of The Cat", who was behind the mask of this feline-oriented superheroine? (4/5)');
if (questionFour.toUpperCase() === 'ICE') {
score++;
}
console.log('score: ' + score);
} else if (randomQuestion === 2) {
// option 2
var questionFour = prompt('Which famous Marvel superhero made a guest appearance in Issue #3 - "Prisoner of War!" (Jan. 1985)? (4/5)');
if (questionFour.toUpperCase() === 'SPIDER-MAN') {
score++;
}
console.log('score: ' + score);
} else {
// option 3
var questionFour = prompt('My first adventures happened way back in World War II. My main enemies were Baron Zemo and The Red Skull. My main weapons were the "Super Soldier" formula, a red, white, and blue shield, and my sidekick, Bucky Barnes. Who am I? (4/5)');
if (questionFour.toUpperCase() === 'CAPTAIN AMERICA') {
score++;
}
console.log('score: ' + score);
}
/* ############################ FIFTH QUESTION ############################ */
//New random number
randomQuestion = Math.floor(Math.random() * 3) + 1;
console.log('random number question 5: ' + randomQuestion);
if (randomQuestion === 1) {
// option 1
var questionFive = prompt("Who is the villain who killed Gwen Stacy, Spider-man's first Love? (5/5)");
if (questionFive.toUpperCase() === 'GREEN GOBLIN') {
score++;
}
console.log('score: ' + score);
} else if (randomQuestion === 2) {
// option 2
var questionFive = prompt('Who is the only person that can travel between both universes? (5/5)');
if (questionFive.toUpperCase() === 'ACCESS') {
score++;
}
console.log('score: ' + score);
} else {
// option 3
var questionFive = prompt('In what Marvel Comics title did the Silver Surfer first start soaring the skies? (5/5)');
if (questionFive.toUpperCase() === 'FANTASTIC FOUR') {
score++;
}
console.log('score: ' + score);
}
console.log('End program');
/* ############################ DISPLAY PLAYER STAR ############################ */
if (score === 5) {
document.write('<h1>You were perfect! 5 stars!</h1>' +
'<div id=stars><p><i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i></p></div>');
} else if (score === 4) {
document.write('<h1>Congratulations! 4 stars!</h1>' +
'<div id=stars><p><i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="far fa-star"></i></p></div>');
} else if (score === 3) {
document.write('<h1>You were good! 3 stars!</h1>' +
'<div id=stars><p><i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i></p></div>');
} else if (score === 2) {
document.write('<h1>Could be better! 2 stars!</h1>' +
'<div id=stars><p><i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i></p></div>');
} else if (score === 1) {
document.write('<h1>At least you tried! 1 stars!</h1>' +
'<div id=stars><p><i class="fas fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i></p></div>');
} else {
document.write('<h1>Try again! 0 stars!</h1>' +
'<div id=stars><p><i class="far fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i> <i class="far fa-star"></i></p></div>');
}
1 Answer

Alexander Solberg
14,350 PointsIt seems you are having a lot of fun :) that's awesome!
What you could maybe pay attention to next time you are coding something like this, is repetition. Particularly the last part of the JavaScript file, there is a lot of html-markup that could simply be stored as a variable and used throughout the conditional.
var resultHtml = '<div id=stars><p><i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i> <i class="fas fa-star"></i></p></div>';
if (score === 5) {
document.write('<h1>You were perfect! 5 stars!</h1>' + resultHtml);
} else if (score === 4) { // etc, etc
Functions would solve most of the repetition and make your code a lot more readable. I assume you haven't reached that part yet, but I'm betting that you'll love it ^^
Leonardo Motta
11,284 PointsLeonardo Motta
11,284 PointsSure, I didn't get to this level yet, just wanted to go a bit further from what was asked, but thanks for your advice!! That is why I posted, but indeed I could create a variable to make it more readable :D